
How to handle boundary error in React
Table of contents
Quick Access
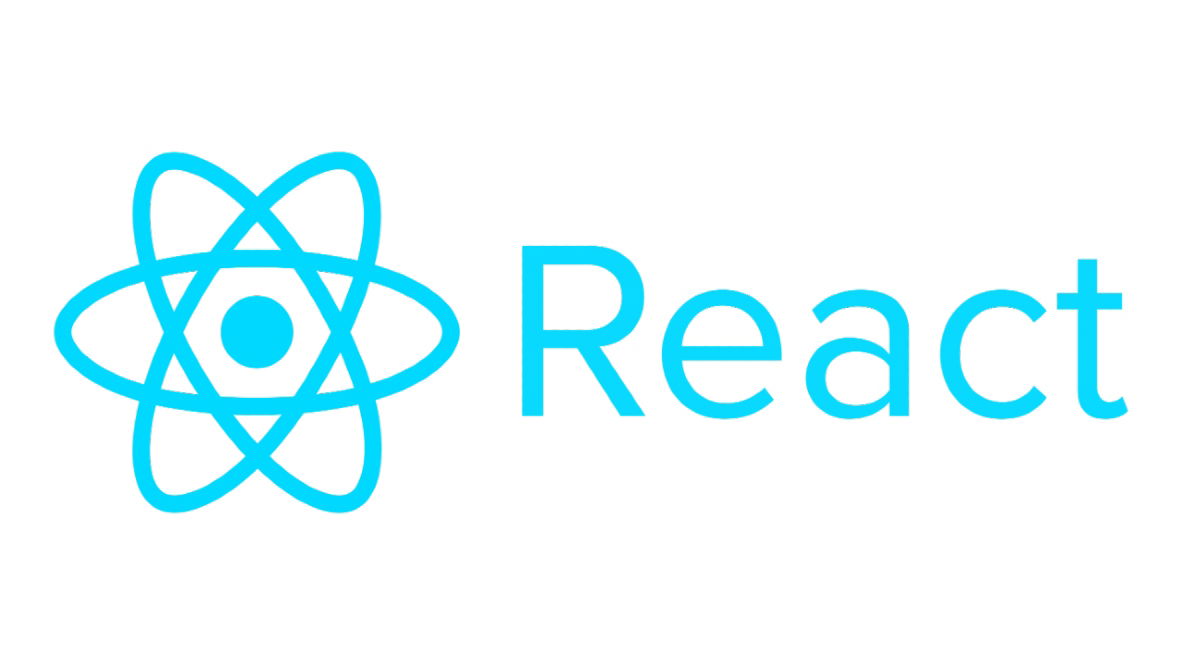
In modern application development with React, ensuring a smooth user experience is essential. Unexpected errors can break the app and leave users with a poor impression.
To mitigate these risks, React offers a robust solution: error boundaries. Keep reading this blog as we explain how to handle error boundaries in React, why they matter, and best practices for implementation.

What is an error boundary in React?
An error boundary is a React component that catches JavaScript errors in its child component tree during:
- The rendering phase.
- Lifecycle methods.
- Component constructors.
When an error is detected, the boundary prevents the entire application from crashing and allows you to display a custom error message or take specific actions, such as logging the error.
Simple error boundary example
import React from 'react';
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state to display the fallback UI
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// You can log the error to an error monitoring service
console.error('Captured error:', error, errorInfo);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
In this example, the ErrorBoundary
component catches errors in its child components and displays a fallback message if an error occurs.
Why should you use error boundaries in React?
Implementing an error boundary in React is essential for several reasons:
- Improves user experience: Prevents a single error from crashing the entire application.
- Facilitates error monitoring: Allows logging errors to services like Sentry or LogRocket.
- Enables customized error responses: You can display user-friendly messages or helpful suggestions.
- Makes code maintenance easier: Errors are easier to identify and fix.
How to implement an error boundary step by step
1. Create the ErrorBoundary Component
Define a class component that implements the getDerivedStateFromError
and componentDidCatch
methods. These are required to detect and handle errors.
2. Wrap vulnerable components
<ErrorBoundary>
<MyVulnerableComponent />
</ErrorBoundary>
3. Display a custom interface
render() {
if (this.state.hasError) {
return (
<div className="error-message">
<h2>Oops! Something went wrong.</h2>
<button onClick={() => window.location.reload()}>Reload</button>
</div>
);
}
return this.props.children;
}
4. Integrate with monitoring services
componentDidCatch(error, errorInfo) {
logErrorToService(error, errorInfo);
}
Best practices for using error boundaries in React
- Place boundaries strategically: Don’t wrap the entire app. Instead, wrap around specific components that are prone to errors.
- Provide friendly messages: Avoid technical jargon in messages for end users.
- Log all errors: Use tools like Sentry to track and analyze errors.
- Offer a recovery action: Provide options like reloading the page or returning to the homepage.
Limitations of error boundaries in React
It’s important to remember that error boundaries cannot catch errors in the following cases:
- Asynchronous errors (such as those in
setTimeout
orPromise
). - Errors occurring outside the React component tree.
- Errors in event handlers controlled by React.
- Errors that occur within the error boundary itself.
For these cases, it’s recommended to use global functions like window.onerror
or window.addEventListener('unhandledrejection')
to catch errors outside of React’s scope.
Managing error boundaries in React is an essential practice for any developer aiming to create robust and reliable applications. By implementing this functionality correctly, you can protect the user experience, facilitate error monitoring, and improve code maintenance.
Don’t underestimate its power: proper error handling can be the difference between a mediocre app and a truly professional one.