
Implementing File Uploads with ReactJS and AWS S3
Table of contents
Quick Access
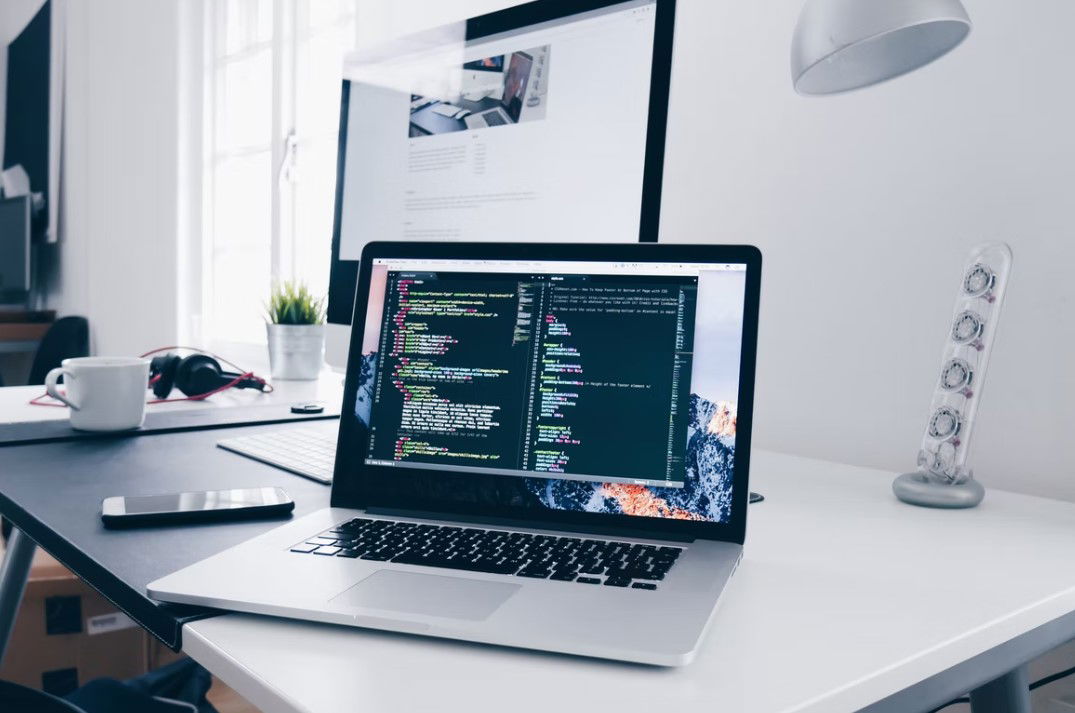
In modern web application development, file management is a common functionality, whether it is for uploading images, documents, or other types of data. One of the most robust and scalable solutions for handling this type of process is AWS S3, Amazon's cloud storage service.
Integrating it with ReactJS not only provides a fast and efficient experience for users, but also ensures that files are stored safely and accessible.
This article will guide you step-by-step in implementing a file upload functionality using ReactJS and AWS S3, explaining the process with practical examples so that any engineer can apply it in their projects.

Why use ReactJS and AWS S3?
ReactJS: Flexibility and performance in the frontend
ReactJS is a widely adopted JavaScript library for building dynamic and efficient user interfaces. Its component-based approach allows you to create fast and scalable web applications, with a clear structure and simplified maintenance.
AWS S3: Secure and Scalable Storage
On the other hand, Amazon S3 (Simple Storage Service) is one of the most reliable and widely used cloud storage services globally. With AWS S3, developers can store large amounts of data securely and with high availability. S3 offers a pay-as-you-go approach, meaning you only pay for the storage you actually use, making it a very attractive option for startups and large companies alike.
The combination of ReactJS to handle the user interface and AWS S3 to manage file storage is an efficient and easy-to-scale solution.
AWS S3 Setup
- Creating a bucket in S3
- Before writing code in ReactJS, we need to prepare our environment in AWS. The first step is to create a "bucket" in S3, which will be where the files will be stored.
- Access the AWS console and navigate to S3.
- Click Create bucket.
- Give your bucket a unique name (the name must be globally unique).
- Select the region in which your bucket will be hosted.
- Configure permissions. Make sure the bucket is set to private, as you'll want to restrict public access to files.
Once the bucket is created, take note of its name and region, as you'll need these in your code.
Setting up access policies
In order for your ReactJS application to be able to upload files, you'll need to create a permissions policy and a user in AWS Identity and Access Management (IAM).
- Create a new user in IAM with programmatic access.
- Assign this user a policy that allows write operations to S3. Make sure the policy allows s3:PutObject on the bucket you just created.
- This setting will allow the application to upload files to S3 without exposing critical credentials.
Implementation in ReactJS
Now that the AWS S3 bucket is ready, it's time to configure the frontend in ReactJS.
Installing Required Libraries
To interact with AWS S3 from ReactJS, we'll be using the official AWS library, AWS SDK, which makes it easy to communicate with AWS services.
In your React project, install the necessary dependencies:
npm install aws-sdk
The AWS SDK will allow you to access AWS services from your React application. You'll also need a library like axios or JavaScript's own fetch to handle HTTP requests in case you choose to sign requests from the backend.
Setting Up the AWS S3 Client in ReactJS
In the React component that will handle file uploads, you must first configure the AWS credentials and the S3 bucket. Avoid exposing your access keys directly on the frontend for security reasons; instead, use a backend to obtain temporary credentials from AWS STS (Security Token Service).
Here's an example of basic file upload configuration:
import React, { useState } from 'react';
import AWS from 'aws-sdk';
const S3_BUCKET = 'your-bucket-name';
const REGION = 'your-region';
AWS.config.update({ accessKeyId: 'YOUR_ACCESS_KEY', secretAccessKey: 'YOUR_SECRET_KEY', region: REGION, });
const myBucket = new AWS.S3({ params: { Bucket: S3_BUCKET }, region: REGION, });
const UploadFileToS3 = () => { const [selectedFile, setSelectedFile] = useState(null);
const handleFileInput = (e) => { setSelectedFile(e.target.files[0]);
};
const uploadFile = (file) => { const params = { ACL: 'public-read', Body: file, Bucket: S3_BUCKET, Key: file.name, };
myBucket.putObject(params) .on('httpUploadProgress', (evt) => { console.log("Loading: ", Math.round((evt.loaded / evt.total) * 100) + '%'); }) .send((err) => { if (err) console.log(err); else console.log("File uploaded successfully"); });
};
return (
<div>
<input type="file" onChange={handleFileInput}/>
<button onClick={() => uploadFile(selectedFile)}>Upload File</button>
</div>
);
};
export default UploadFileToS3;
Code Explanation
- AWS SDK Configuration: We update the AWS configuration with the bucket credentials and region.
- File Selection: The selectedFile state manages the file that the user selects in the input.
- Upload to S3: The putObject method of S3 allows you to upload the selected file to the S3 bucket. While the file is uploading, we show a progress in the console.
File Upload Security
It is important to note that AWS access credentials should not be present in the frontend. This would compromise security. of your application. It is recommended to use temporary credentials or implement URL signing in the backend.
To do this, you can use AWS Amplify, which securely handles authentication and file upload operations, or set up a Node.js backend that takes care of signing upload URLs.
Implementing a file upload functionality using ReactJS and AWS S3 is a relatively simple, yet very powerful process. Not only does it allow applications to handle files efficiently, but it also integrates with AWS's scalable and secure infrastructure.
If you want to implement custom file upload solutions or improve your cloud storage infrastructure, Rootstack can help you design and implement the best solution tailored to your needs.
Related blogs
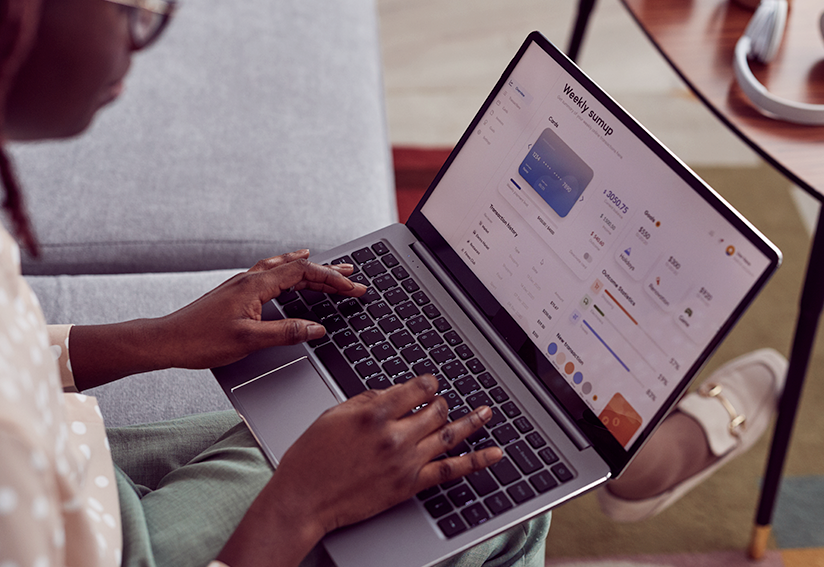
BPM Financial Services: Key Benefits for Your Business
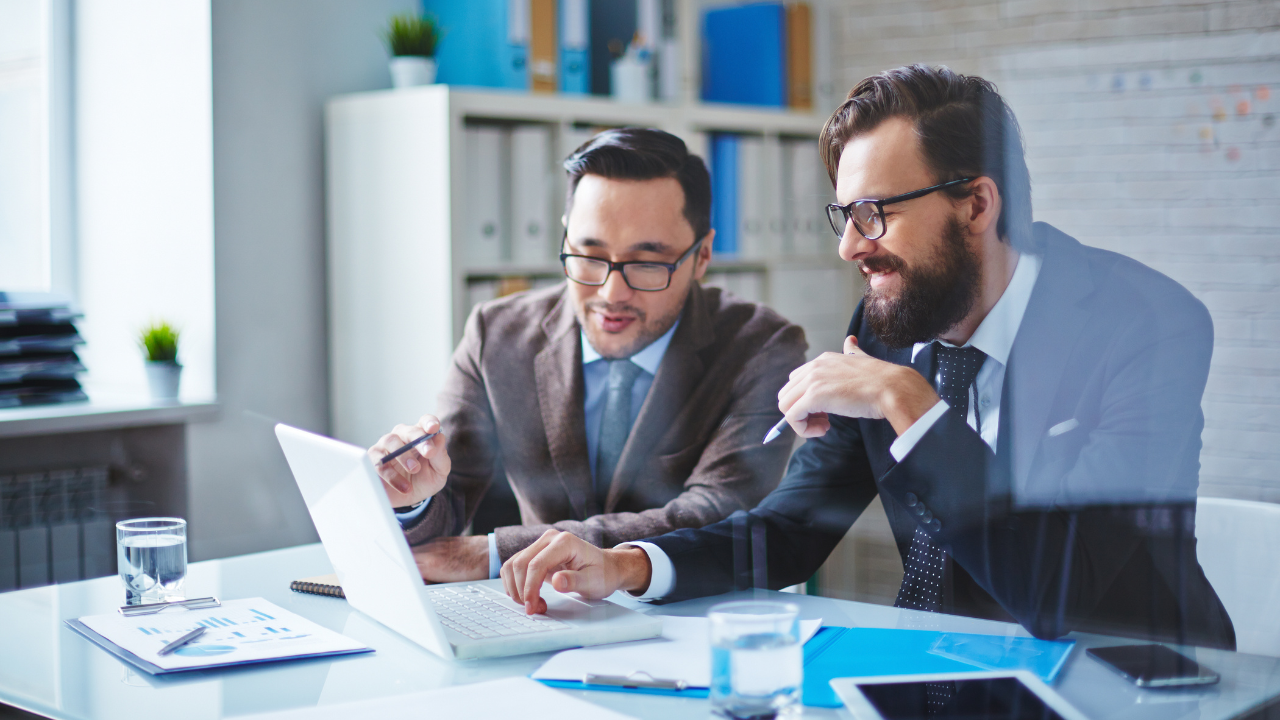
Generative AI consulting services: How to make your business more profitable
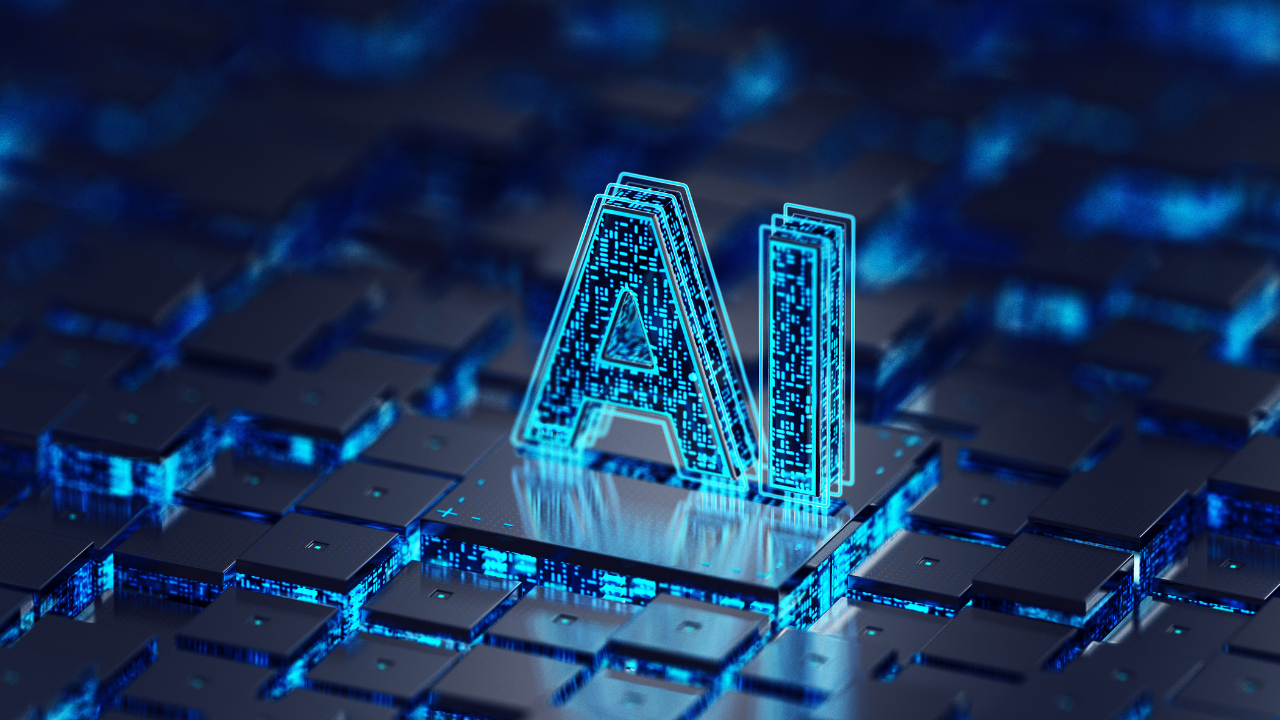
Generative AI use cases: 6 industries that benefit from this technology
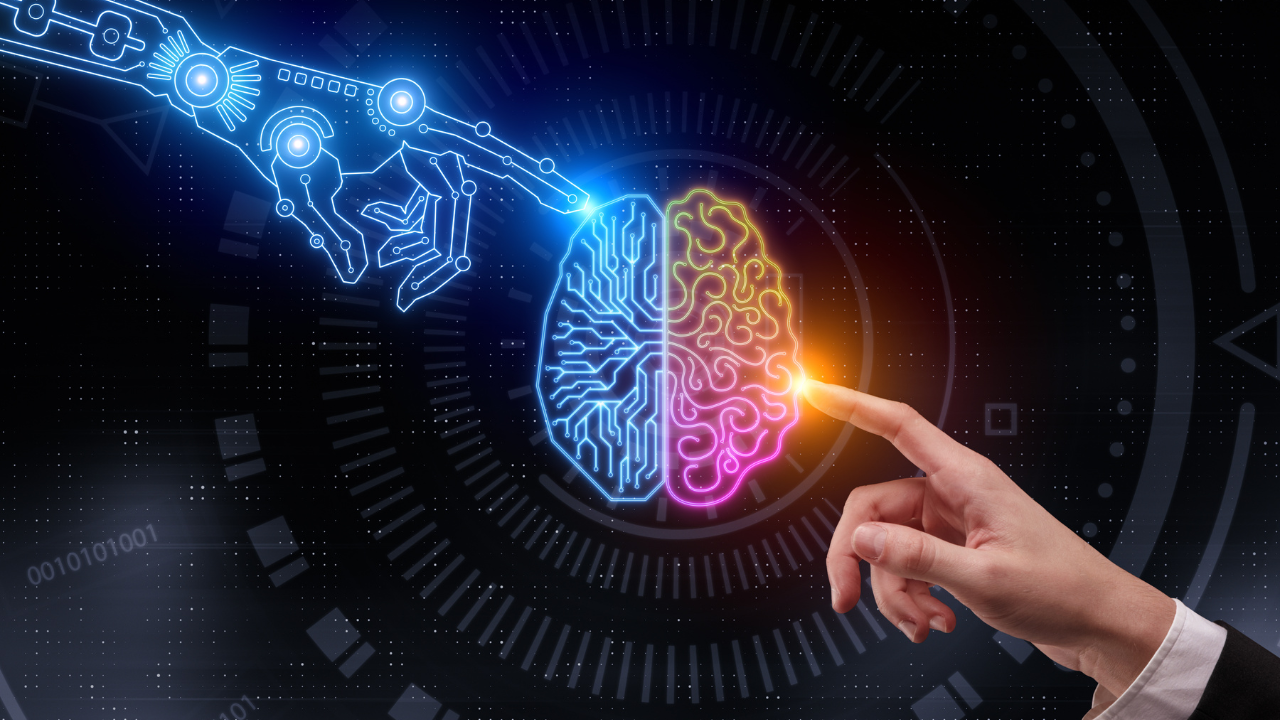
Generative AI vs AI: Similarities, differences, and ethical implications
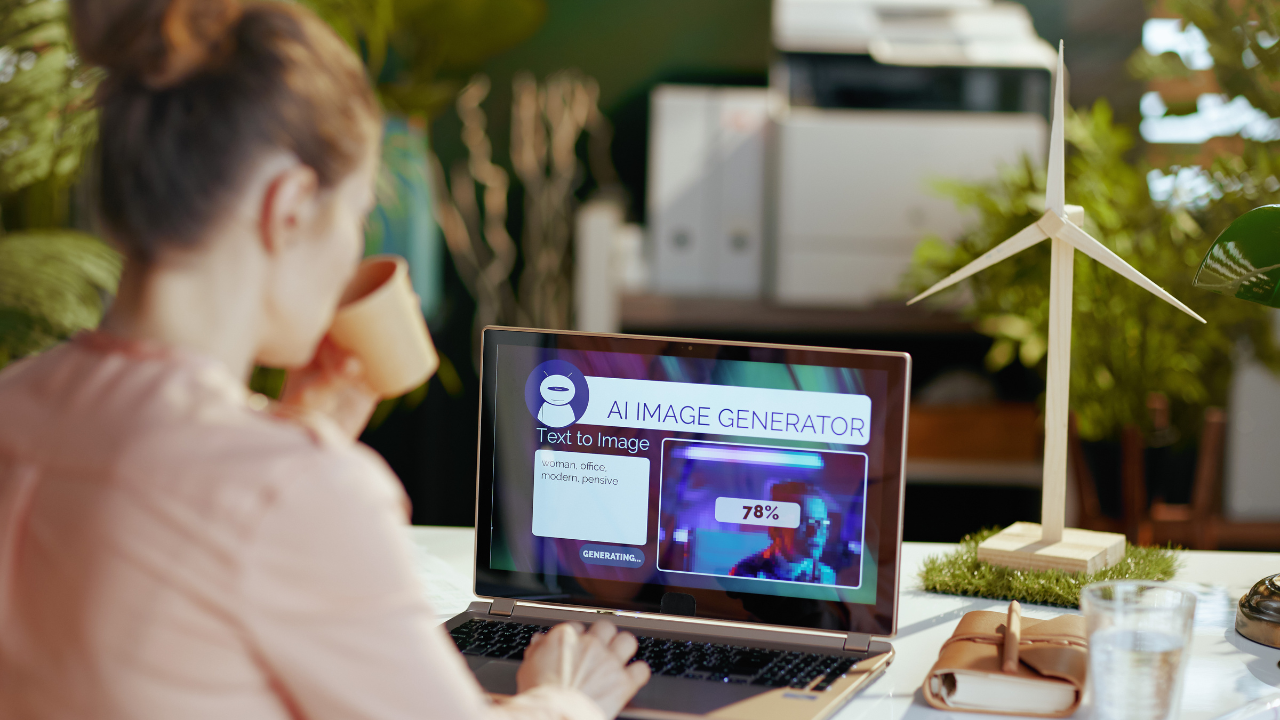
What is generative AI? How it works, implementation, and examples
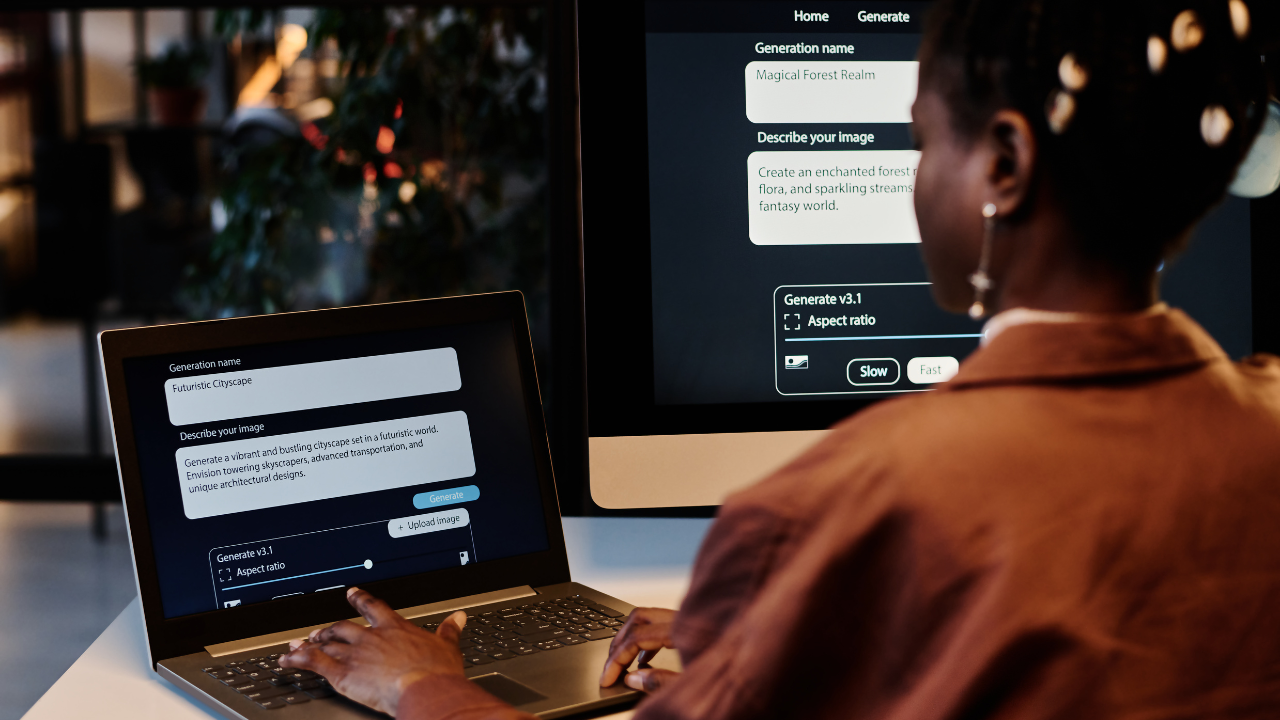