
Node.js Streams: Deep Dive and Practical Applications
Table of contents
Quick Access
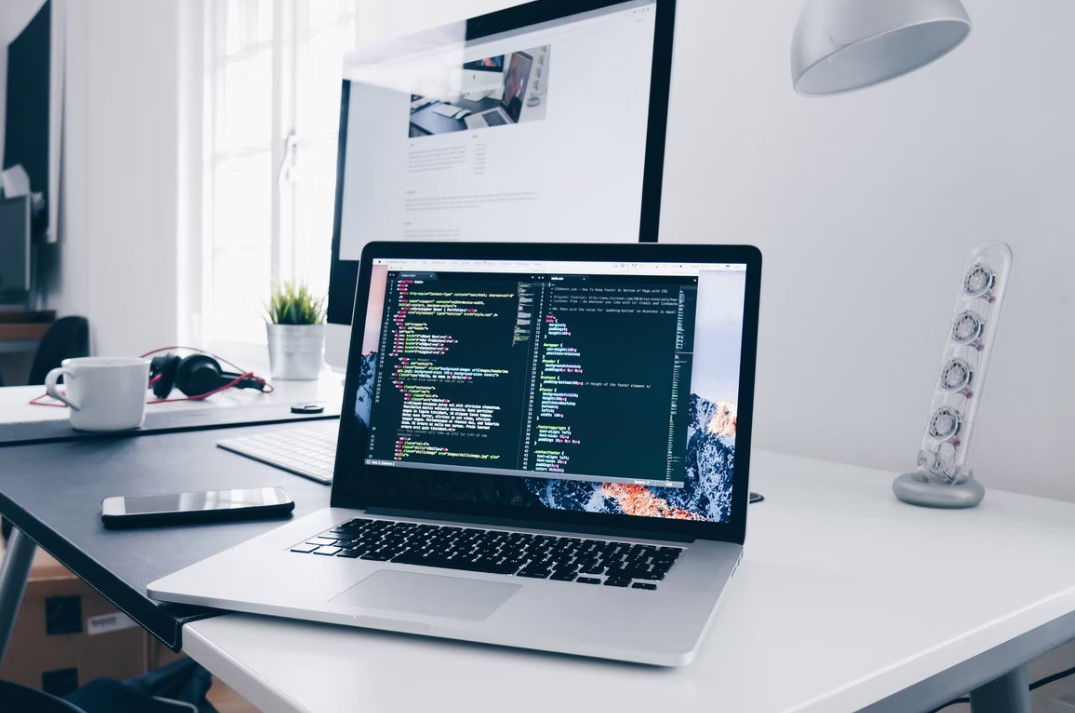
Node.js is an extremely popular platform for developing JavaScript-based applications, especially for its focus on efficient handling of asynchronous input/output (I/O). One of the most powerful and least understood components of Node.js is streams. Node.js streams allow you to process large amounts of data efficiently and in real-time, without having to load the entire content into memory.
In this article, we're going to dive deeper into what streams are in Node.js, how they work, and the practical applications they can have in real-world projects. If you already have some prior knowledge of Node.js but don't yet fully understand how streams work, this guide will provide you with a clear and useful technical analysis.
What are Streams in Node.js?
A stream is simply a collection of data that moves from one place to another in a continuous flow, rather than loading the entire data set at once. Streams are ideal for processing large files, working with networks, or handling data in real time, as they can process parts of a file or input without waiting for the entire load to complete.
Node.js offers four main types of streams:
- Readable Streams: allow you to read data from a source.
- Writable Streams: allow you to write data to a destination.
- Duplex Streams: combine the features of readable and writable, allowing you to read and write simultaneously.
- Transform Streams: are duplex streams that allow you to modify data while reading or writing.
These streams are primarily used to manipulate data that flows through the application, such as files, buffers, or even HTTP requests.

Advantages of Using Streams in Node.js
Using streams offers several key advantages, particularly in situations where large volumes of data or intensive input/output are being handled:
- Memory efficiency: By processing data in small chunks, you avoid loading large files or data streams into memory, which improves application performance.
- Performance: Streams allow you to start processing data as soon as it's available, meaning you can operate on data in real time without waiting for the entire data to finish loading.
- Scalability: Streams are perfect for applications that need to handle multiple simultaneous streams of data, such as web servers that handle many HTTP requests.
In short, streams are ideal for situations where large amounts of data are being handled or fast responses are required.
Types of Streams in Detail
Readable Streams
A Readable Stream is a stream from which data can be read. This data is received in chunks. A common example of a Readable Stream in Node.js is reading files using the fs module:
const fs = require('fs');
const readableStream = fs.createReadStream('file.txt', { encoding: 'utf8' });
readableStream.on('data', (chunk) => {
console.log('New chunk received:', chunk);
});
This code shows how to efficiently read a file in chunks instead of loading the entire file into memory. Each chunk is processed independently, allowing memory to be freed up for other processes.
Writable Streams
On the other hand, Writable Streams allow you to write data sequentially. A classic example would be writing data to a file:
const writableStream = fs.createWriteStream('output.txt');
writableStream.write('This is the first chunk of data.\n');
writableStream.write('This is the second chunk of data.\n');
writableStream.end();
Using writable streams is essential for handling outgoing data without needing to buffer it completely in memory before sending it.
Duplex Streams
A Duplex Stream combines both read and write capabilities. A common example of this is a network socket, where data can be sent and received simultaneously:
const net = require('net');
const server = net.createServer((socket) => {
socket.write('Connection established.\n');
socket.on('data', (data) => {
console.log('Data received:', data.toString());
});
});
server.listen(8080);
This example shows how you can create a server that communicates over sockets using read and write streams at the same time.
Transform Streams
Transform streams allow you to modify data while it is being read or written. They are especially useful when you need to apply transformations to the data, such as compression or encryption. For example, the zlib module in Node.js allows you to compress files using transform streams:
const zlib = require('zlib');
const fs = require('fs');
const gzip = zlib.createGzip();
const readable = fs.createReadStream('input.txt');
const writable = fs.createWriteStream('input.txt.gz');
readable.pipe(gzip).pipe(writable);
This example compresses a file using the pipe() method, which is a way to connect multiple streams and create data processing pipelines.
Practical Applications of Node.js Streams
1. File Streaming
One of the most common uses of streams in Node.js is efficient handling of large files. Instead of loading an entire file into memory, which could cause performance issues, you can use a readable stream to read chunks and process them gradually.
2. Video or Audio Streaming
In multimedia applications, streams are critical to enabling real-time streaming. You can start playing a portion of an audio or video file while the rest of the file is still downloading.
3. Real-Time Data Processing
Another useful application is real-time data processing, such as handling large incoming streams of data from sensors or IoT devices. By using streams, you can analyze or modify the data without having to store it all.
Streams in Node.js are a powerful tool for efficiently handling data, whether you're working with files, network connections, or large amounts of real-time data. Understanding how different types of streams work and their practical applications can dramatically improve your application's performance and reduce memory consumption.
If you're looking to implement Node.js-based solutions or need to optimize your infrastructure to handle large volumes of data, our agency can help you get the most out of the most advanced technologies. Contact us to learn more about how we can integrate Node.js-based streams solutions into your next project.
We recommend you on video
Related blogs
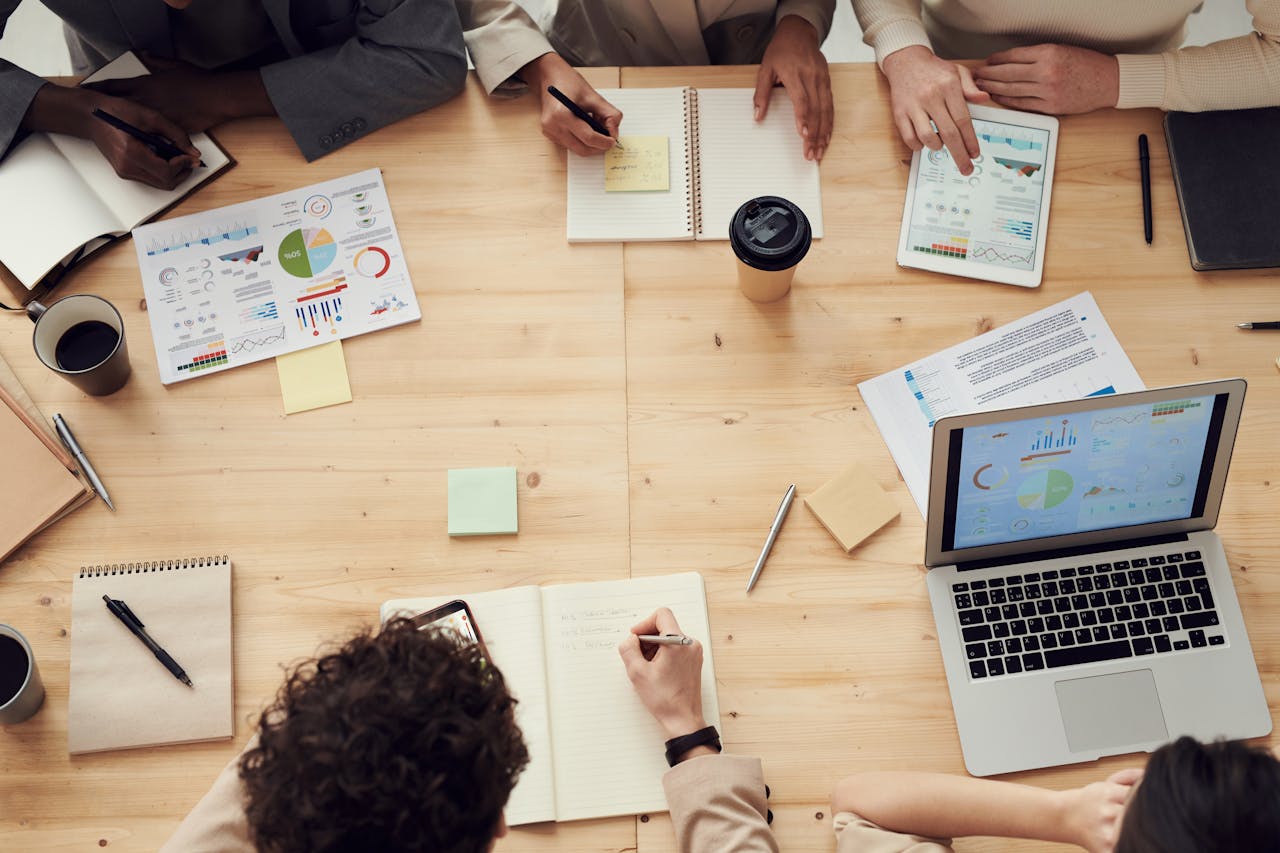
Redefining Teamwork with AI: What's New in Jira, Confluence, and Bitbucket in 2025
What is a consultation software?
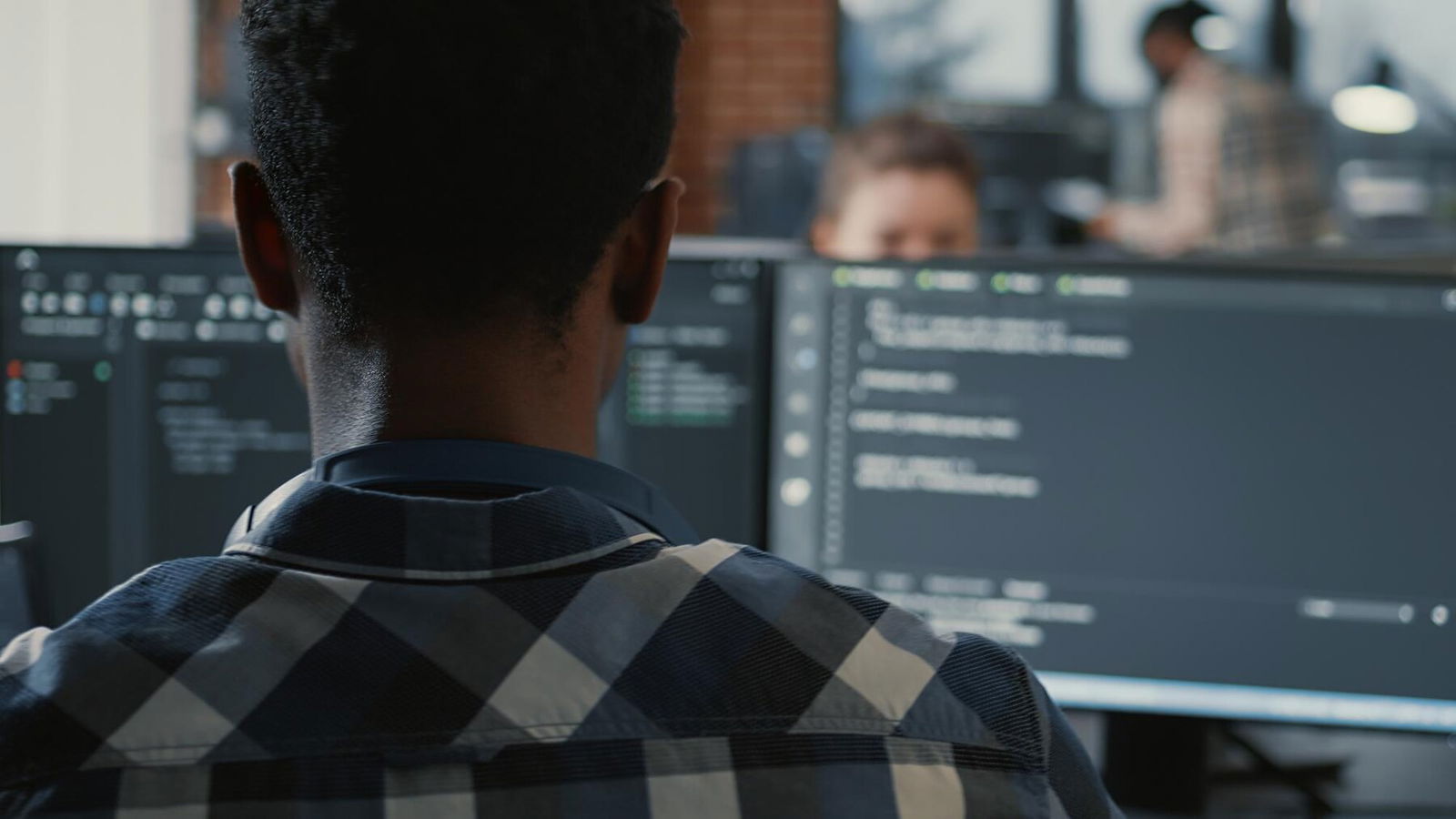
ERP Software Consultant: Rootstack is the option you need

Modern core banking: Building seamless Front-End to Back-End communication
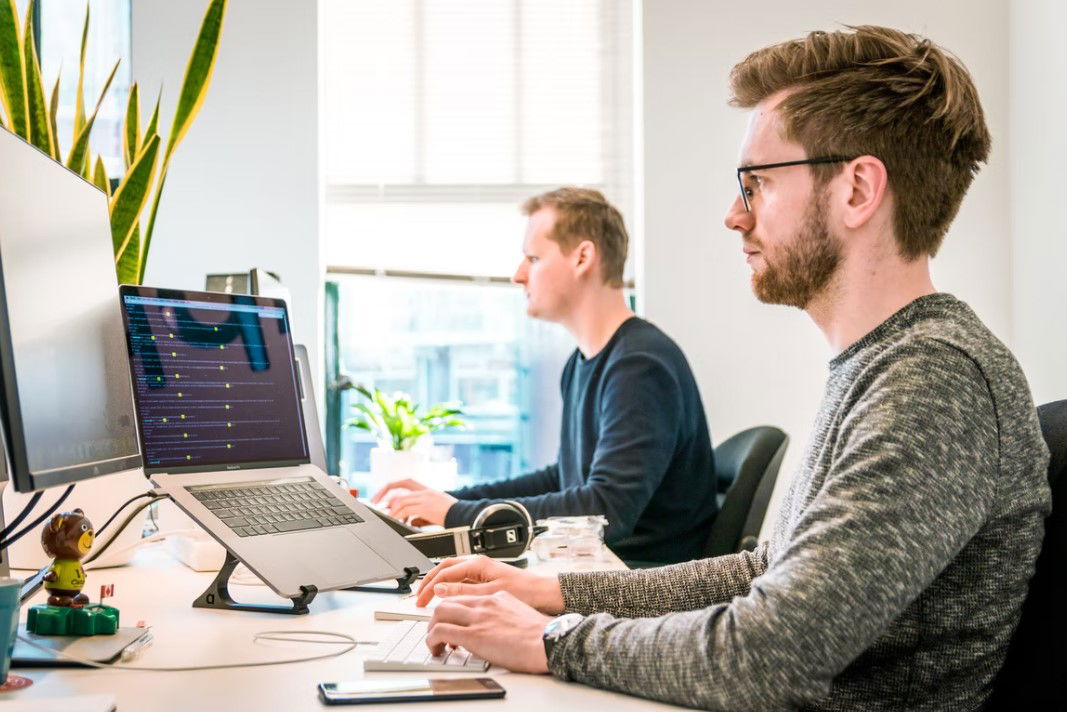
Reasons why you need a software architecture consultancy
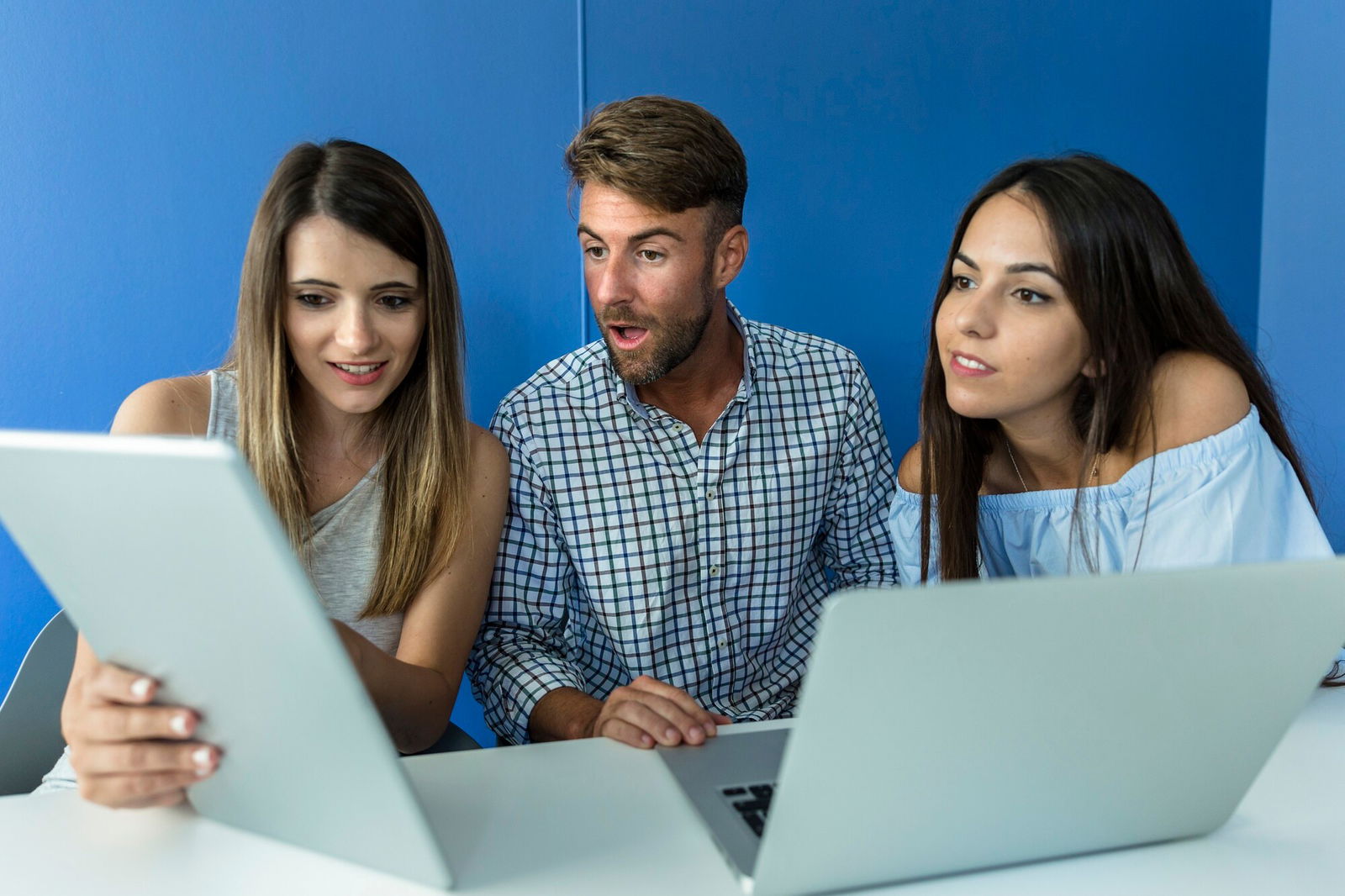