
How do JavaScript functions work?
Table of contents
Quick Access
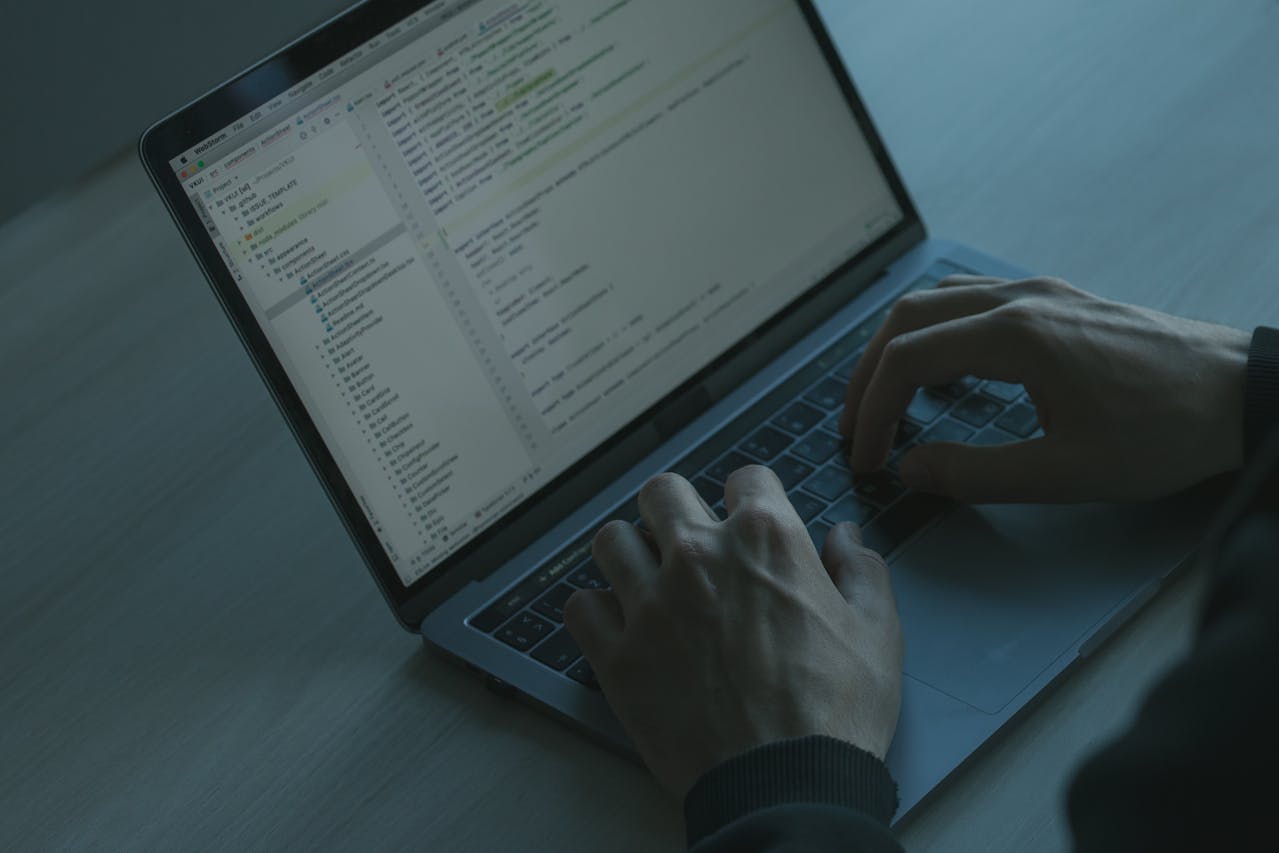
JavaScript functions are a fundamental aspect of the language and allow developers to write modular, reusable, and organized code. Understanding how JavaScript functions work is essential for both novice and experienced programmers.
Need a refresher? Let's see how they explain JavaScript functions in MDM Web Docs “A function in JavaScript is similar to a procedure: a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it must take some input and return an output where there is some obvious relationship between the input and the output. To use a function, you must define it somewhere in the scope from which you want to call it.”
This article explores the core concepts, types, and mechanisms behind JavaScript functions.

What is a JavaScript function?
Explaining in more detail, a function in JavaScript is a block of code designed to perform a particular task. Functions are executed when they are invoked (called). JavaScript functions are first-class citizens, meaning they can be treated like any other variable, passed as arguments to other functions, and returned from other functions.
Defining a function
There are several ways to define functions in JavaScript:
Function declaration:
function greet(name) {
return `Hello, ${name}!`;
}
Function expression:
const greet = function(name) {
return `Hello, ${name}!`;
};
Arrow function (ES6+):
const greet = (name) => `Hello, ${name}!`;
Anonymous function
setTimeout(function() {
console.log('This runs after 1 second');
}, 1000);
Invoke functions
To execute a function, it must be invoked. This is done by adding parentheses to the function name and optionally passing arguments:
greet('Alice'); // Outputs: Hello, Alice!
Parameters and arguments
Functions can take parameters, which are specified when the function is defined, and arguments, which are the actual values passed to the function when it is invoked.
function sum(a, b) {
return a + b;
}
sum(2, 3); // Outputs: 5
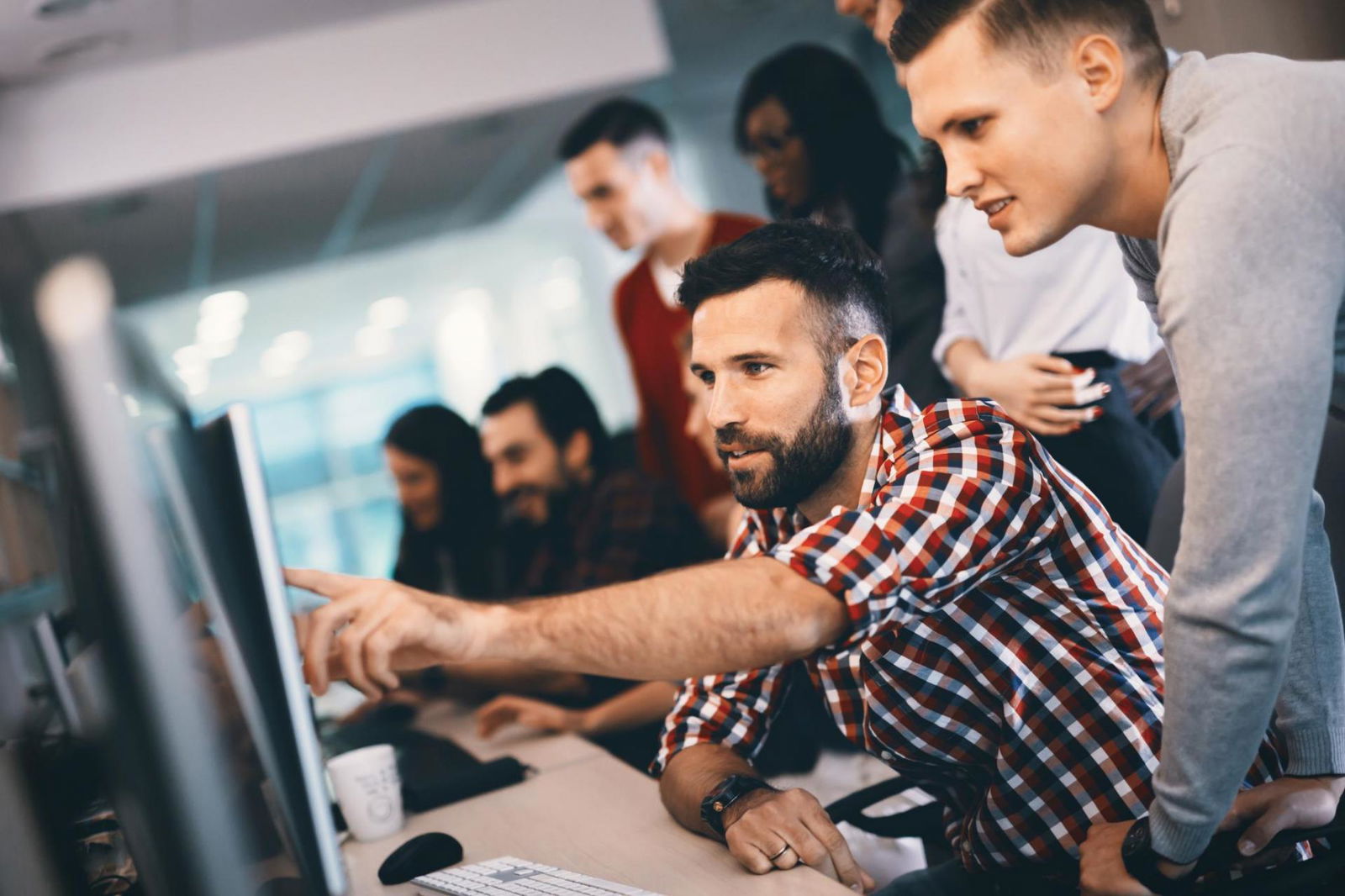
Return values
Functions can return values using the return statement. If no return statement is used, the function returns undefined by default.
function multiply(a, b) {
return a * b;
}
let result = multiply(4, 5); // result is 20
Scope and closures
The scope determines the accessibility of the variables. JavaScript is function-scoped, meaning that variables declared inside a function are not accessible outside of it.
function example() {
let x = 10;
console.log(x); // 10
}
console.log(x); // ReferenceError: x is not defined
Closures occur when a function retains access to its lexical scope, even when the function is executed outside that scope.
function outerFunction() {
let outerVariable = 'I am outside!';
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const inner = outerFunction();
inner(); // Outputs: I am outside!
Higher order functions
Functions that take other functions as arguments or return them as output are called higher-order functions. This is a powerful feature of JavaScript that enables functional programming paradigms.
function higherOrderFunction(callback) {
callback();
}
function sayHello() {
console.log('Hello!');
}
higherOrderFunction(sayHello); // Outputs: Hello!
Immediately Invoked Function Expressions (IIFE)
An IIFE is a function that is executed immediately after its definition. This pattern is often used to create a new scope and avoid polluting the global namespace.
(function() {
console.log('This function runs immediately!');
})();
Asynchronous functions
With the advent of ES6, JavaScript introduced promises and async/await to handle asynchronous operations more elegantly.
Using promises:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Data fetched');
}, 2000);
});
}
fetchData().then(data => console.log(data)); // Outputs: Data fetched after 2 seconds
Using async/await:
async function fetchData() {
const data = await new Promise((resolve) => {
setTimeout(() => {
resolve('Data fetched');
}, 2000);
});
console.log(data);
}
fetchData(); // Outputs: Data fetched after 2 seconds
JavaScript functions are versatile and powerful, providing the building blocks for creating complex applications. By mastering the features, developers can write clean, maintainable, and efficient code. Whether it's simple utilities or complex asynchronous operations, understanding how functions work is key to realizing the full potential of JavaScript.
We recommend you on video
Related blogs

AWS Architecture for Online Banking
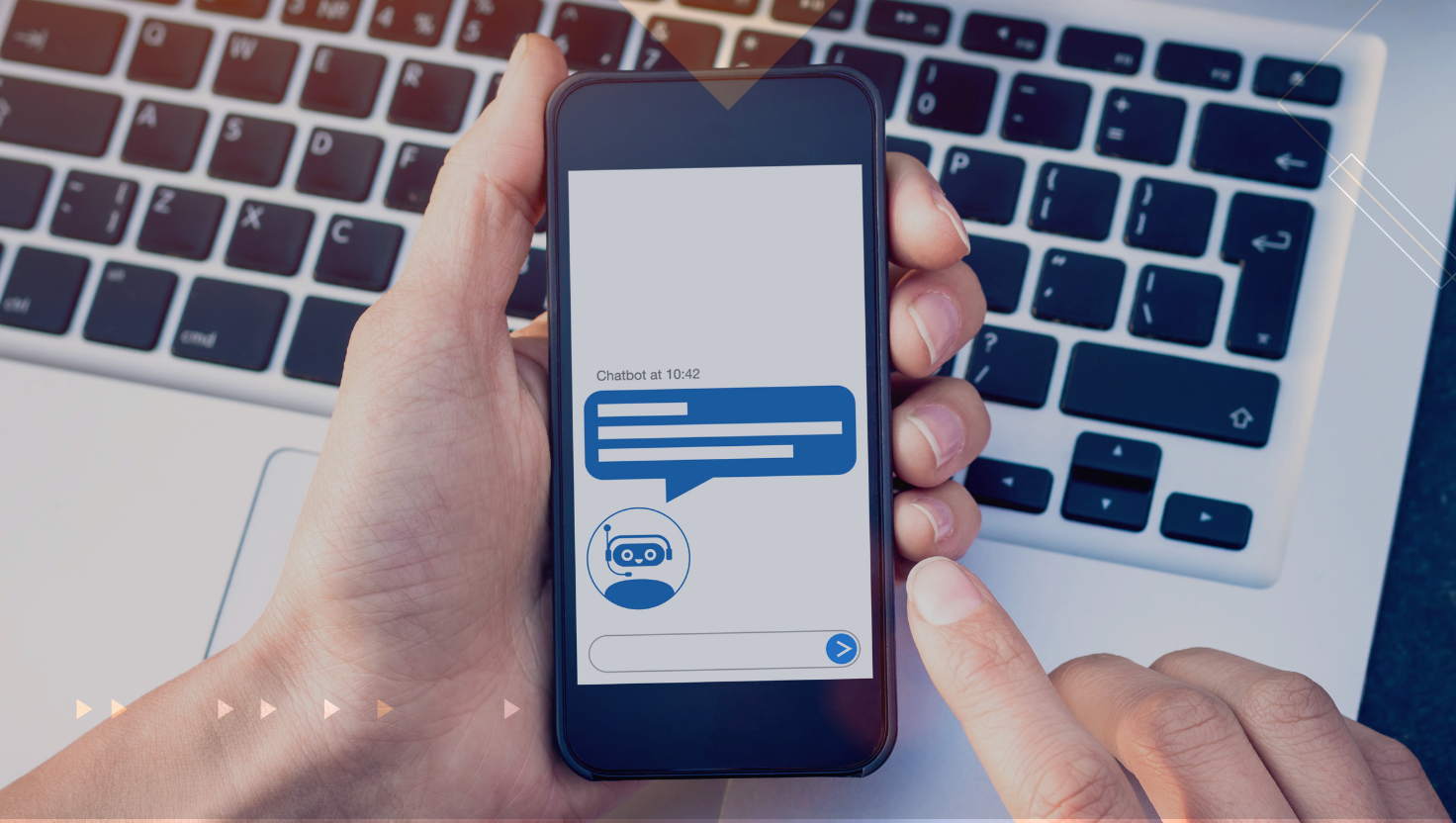
How Rootstack's intelligent chatbots are transforming businesses' operational efficiency
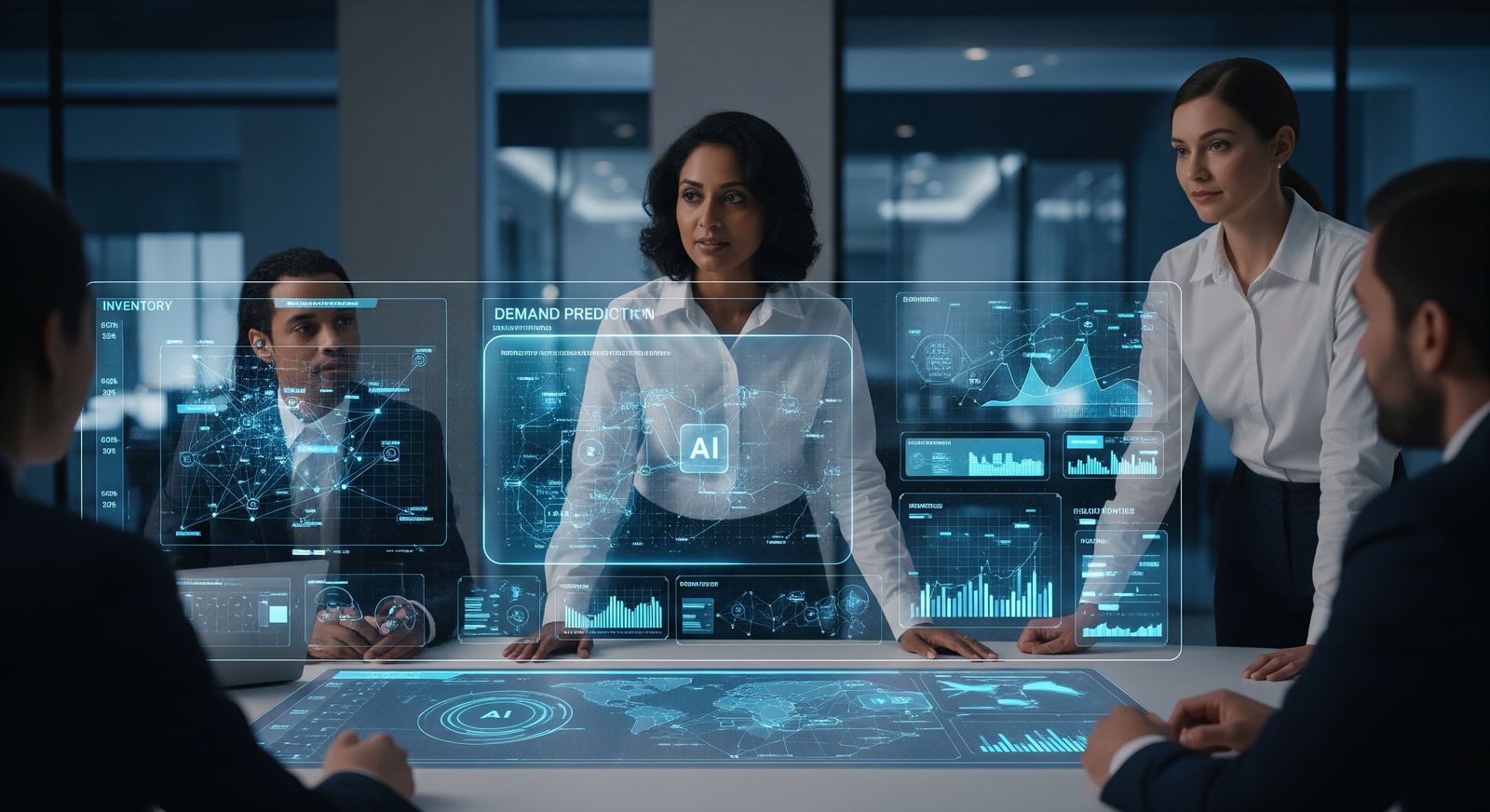
Data Integration: the key link to a solid Business Intelligence architecture
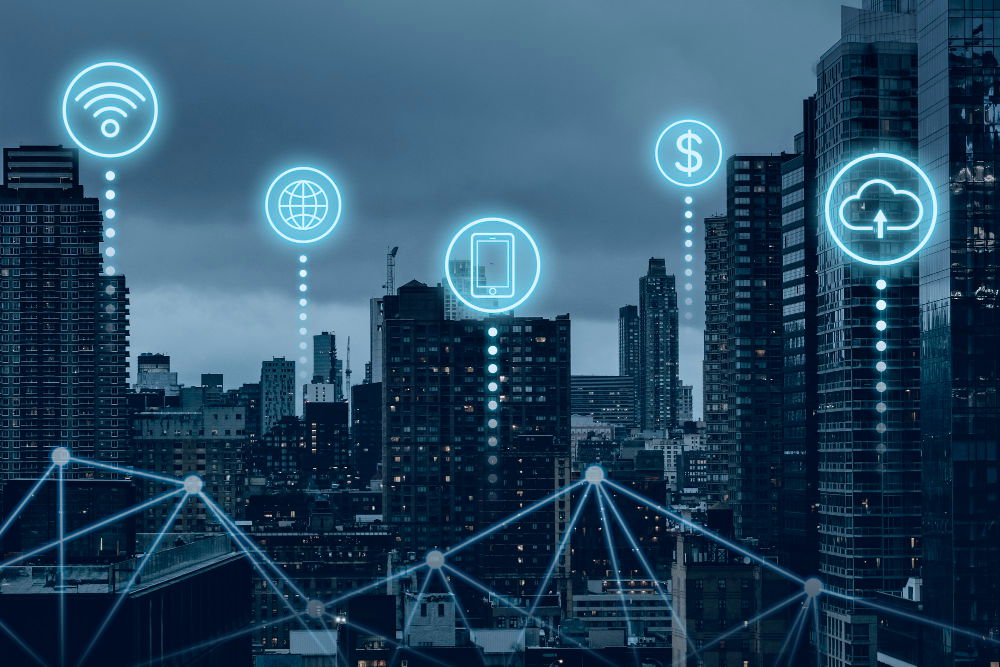
What is Business Intelligence and how to implement it: Step by step
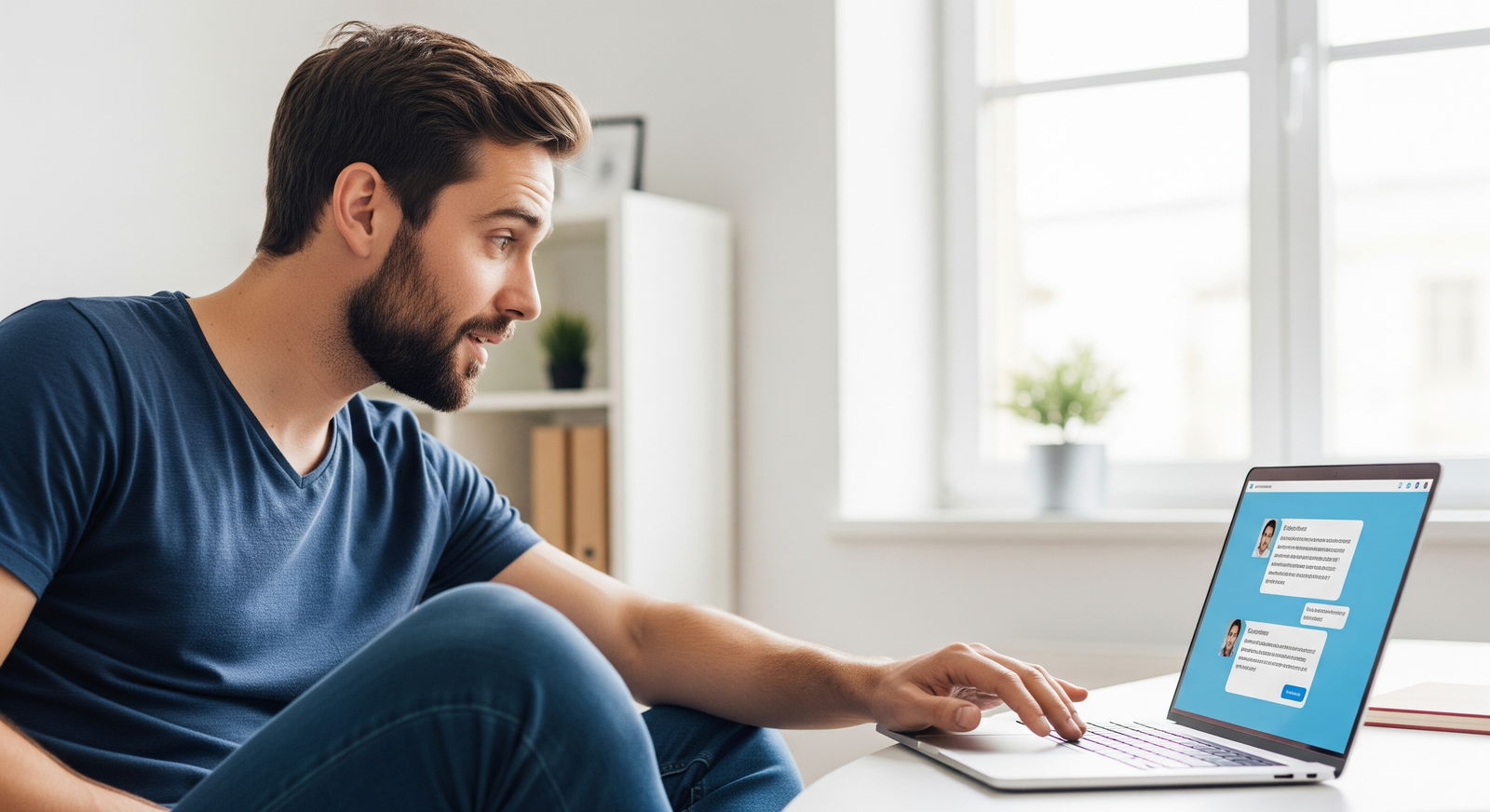
How to integrate a chatbot into a website: A guide for business leaders
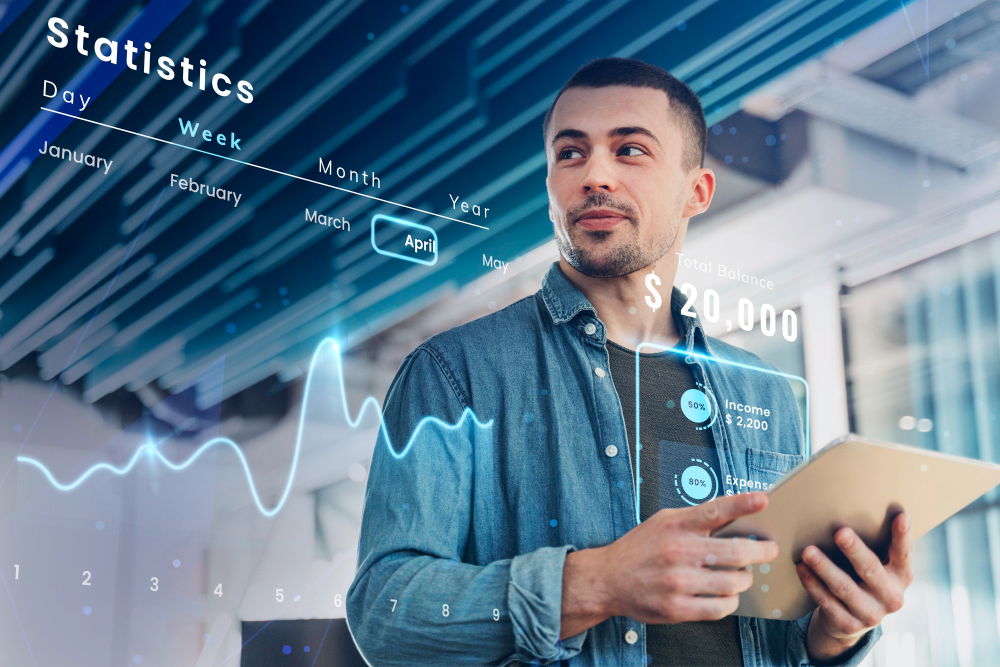