
Speeding up your app's build with Vite and SWC
Table of contents
Quick Access
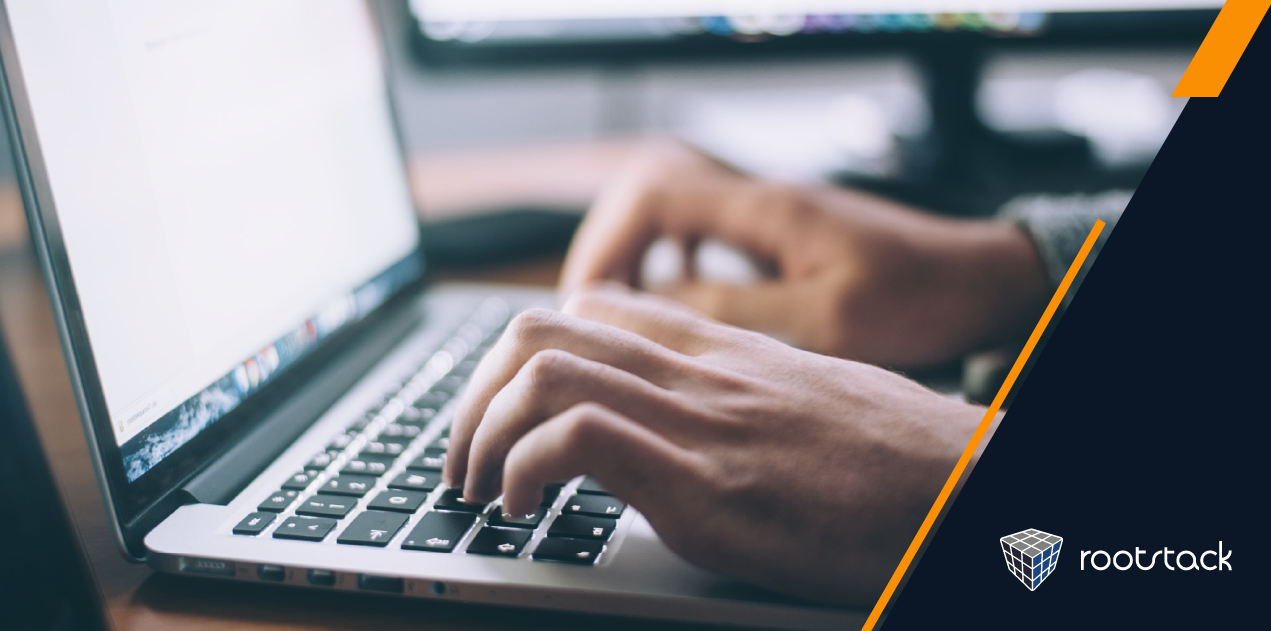
If you are looking for alternative ways to build and bundle your Javascript or Typescript app, you should consider using Vite. Vite is a new alternative to other build tools such as Webpack, Parcel, and Gulp.js. It aims to leverage newer web technologies such as Javascript Modules while also requiring minimal configuration in comparison to its competitors.
Vite is designed to be extensible through its own plugin system, and currently has support for most JavaScript frameworks and ecosystem tools, including community favorites such as React, Vue, Svelte, and many more.
Vite can be an all-in-one solution for all of the following:
- Compiling from another language to native JavaScript, such as TypeScript
- Compiling JavaScript with special language add-ons, such as JSX
- Compiling additional non-JavaScript languages, such as SCSS
- Compiling with support for specific ECMA versions
- Starting a development server with hot reloading
- Code splitting and modularization
- Bundling and minification
- Introduction to Vite
Vite is available as the NPM package vite. Getting started with Vite can be as simple as installing it as a development dependency in an existing project using your preferred package manager:
npm install --save-dev vite
Vite configuration is located in vite.config.js and is highly minimal by default. For a standard project without any additional JavaScript technology, the configuration file could look like this:
import { defineConfig } from 'vite';
export default defineConfig({})
Vite will build our app using the entry point file ./src/main.js (or main.ts, main.tsx, etc. depending on preferred language), and create output files inside dist.
Vite offers three main CLI commands, which you can add to your project's scripts:
- vite: start the development server (with hot reloading)
- vite build: build the app and write files to dist
- vite preview: serve files built with the build command
If building an app for the browser, create an index.html file, and Vite will also include it in the dist output.
We can add plugins to extend Vite's default compilation capabilities. For example, if we wanted to add support for React with JSX:
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react'; // make sure to install this as a dev dependency
export default defineConfig({
plugins: [react()]
})
Vue's bundling functionality is based on Rollup, which may be also be configured directly for advanced use cases. See the documentation for more information.
Using TypeScript
Vite also offers the option to write in alternative languages. TypeScript is supported by default thanks to its popularity as an alternative to JavaScript.
In order to write your app in TypeScript, simply install the typescript package as a dev dependency, create the TypeScript configuration files as needed, and write your Vite configuration to a TypeScript file named vite.config.ts instead of a JavaScript file.
More specifically, Vite recommends creating three tsconfig files:
- a main config file (tsconfig.json)
- configuration for compiling your app for the browser (tsconfig.app.json)
- configuration for compiling Vite's processes, which are run locally with Node (tsconfig.node.json)
See the recommended TS configuration files for a React app here:
tsconfig.json
tsconfig.app.json
tsconfig.node.json
Using the Vite creation script
When creating a new project, you may use Vite's official creation script, easily accessed with the npm create command. For example, to create a new Vite app with no specific template or name:
npm create vite@latest
You may also run the command with a specific details if already available:
npm create vite@latest my-app -- --template react
You can use this command to quickly create example projects for your desired technology stack instead of writing config from scratch.

Adding SWC
SWC stands for Speedy Web Compiler. SWC is an extensible set of build tools written in Rust. SWC compilation very often performs better than when using similar tools (such as Babel). Some Vite build plugins, such as @vitejs/plugin-react, may optionally be replaced with an SWC-based equivalent in order to achieve very quick compilation, and, by extension, a faster-loading development server. In some cases, it may also produce even smaller output files.
If writing a React app, we could simply change our plugins in the Vite config to use SWC:
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react-swc'; // notice that this dependency changes.
export default defineConfig({
plugins: [react()]
})
If using the npm create script you will notice that some templates also offer variants with SWC. Note that SWC may still be missing support for certain languages.
Related blogs
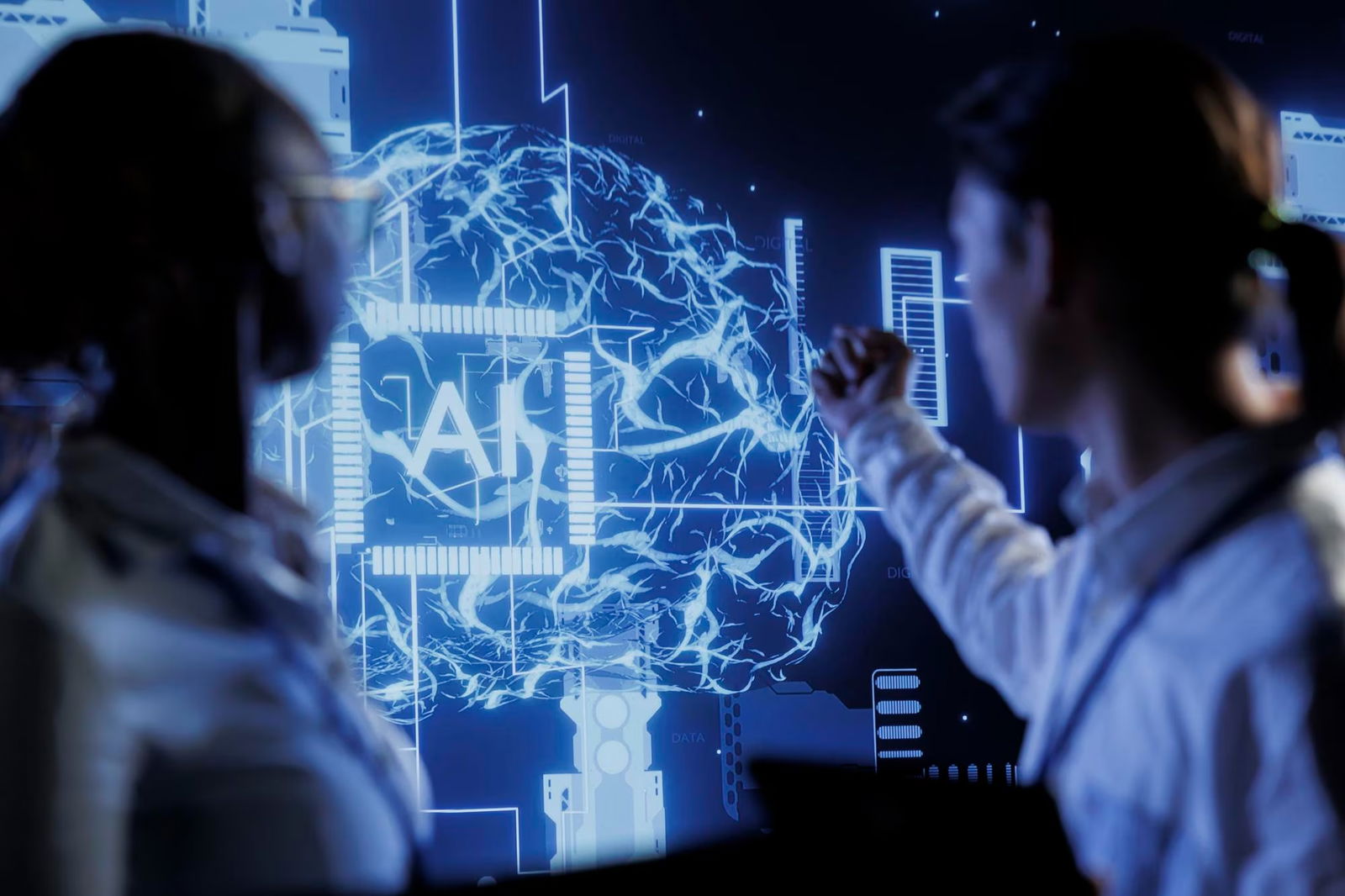
How banks are saving millions with AI-powered customer service
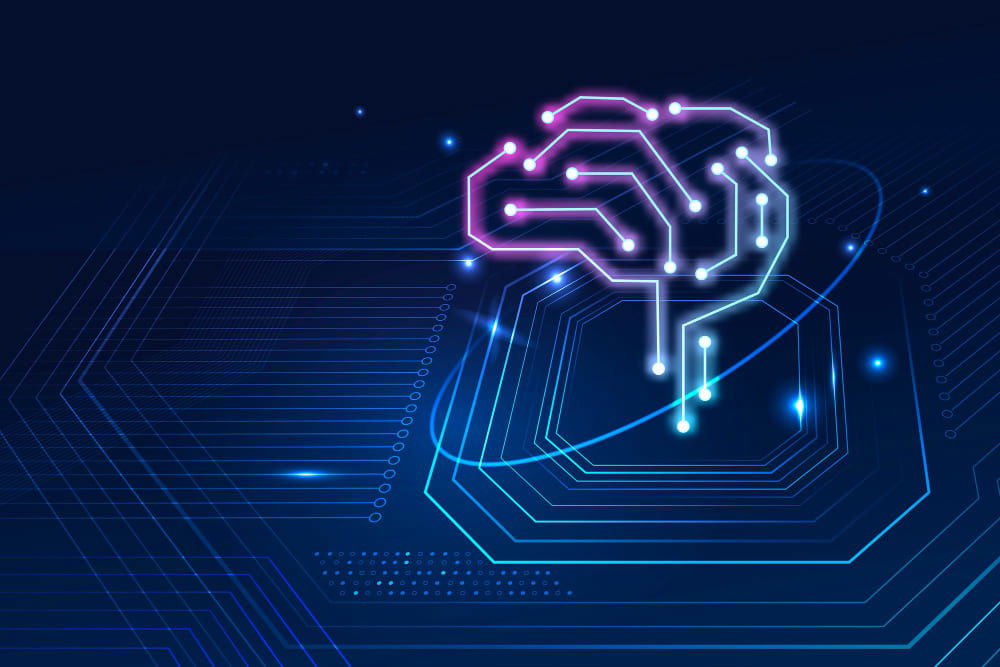
Statistics on the use and effectiveness of Machine Learning solutions
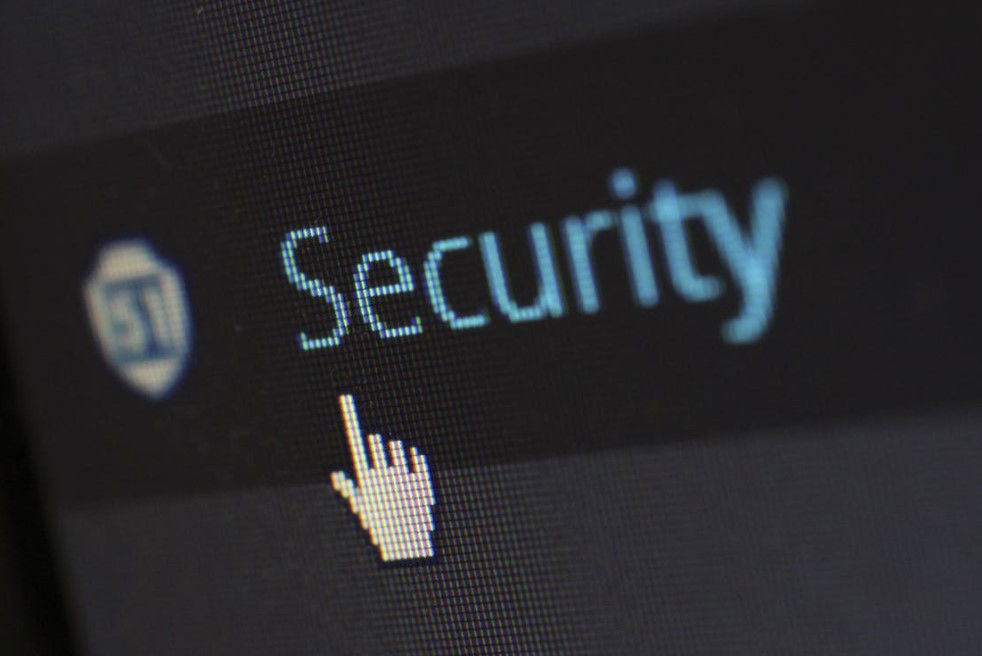
How AI is Revolutionizing Fraud Detection in Banking
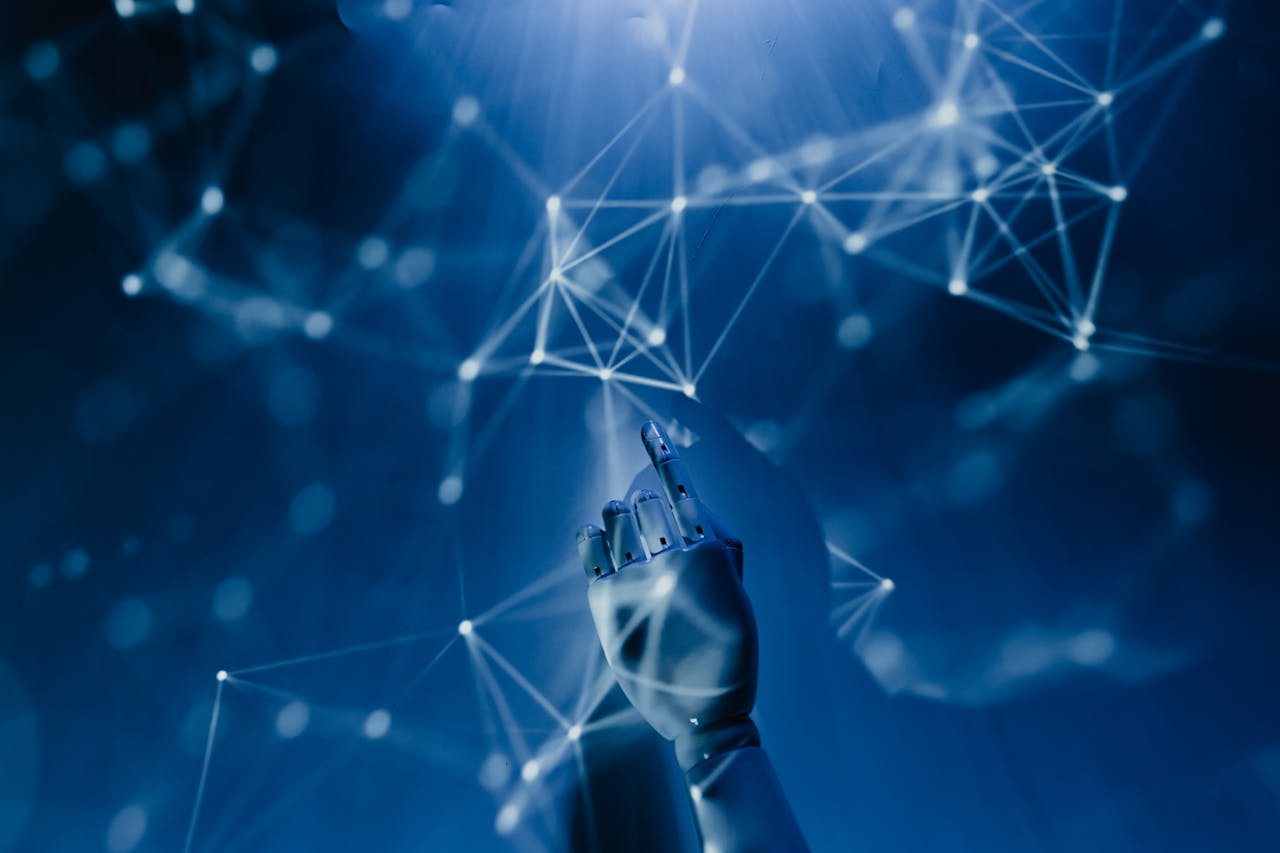
How machine learning is transforming banking and finance
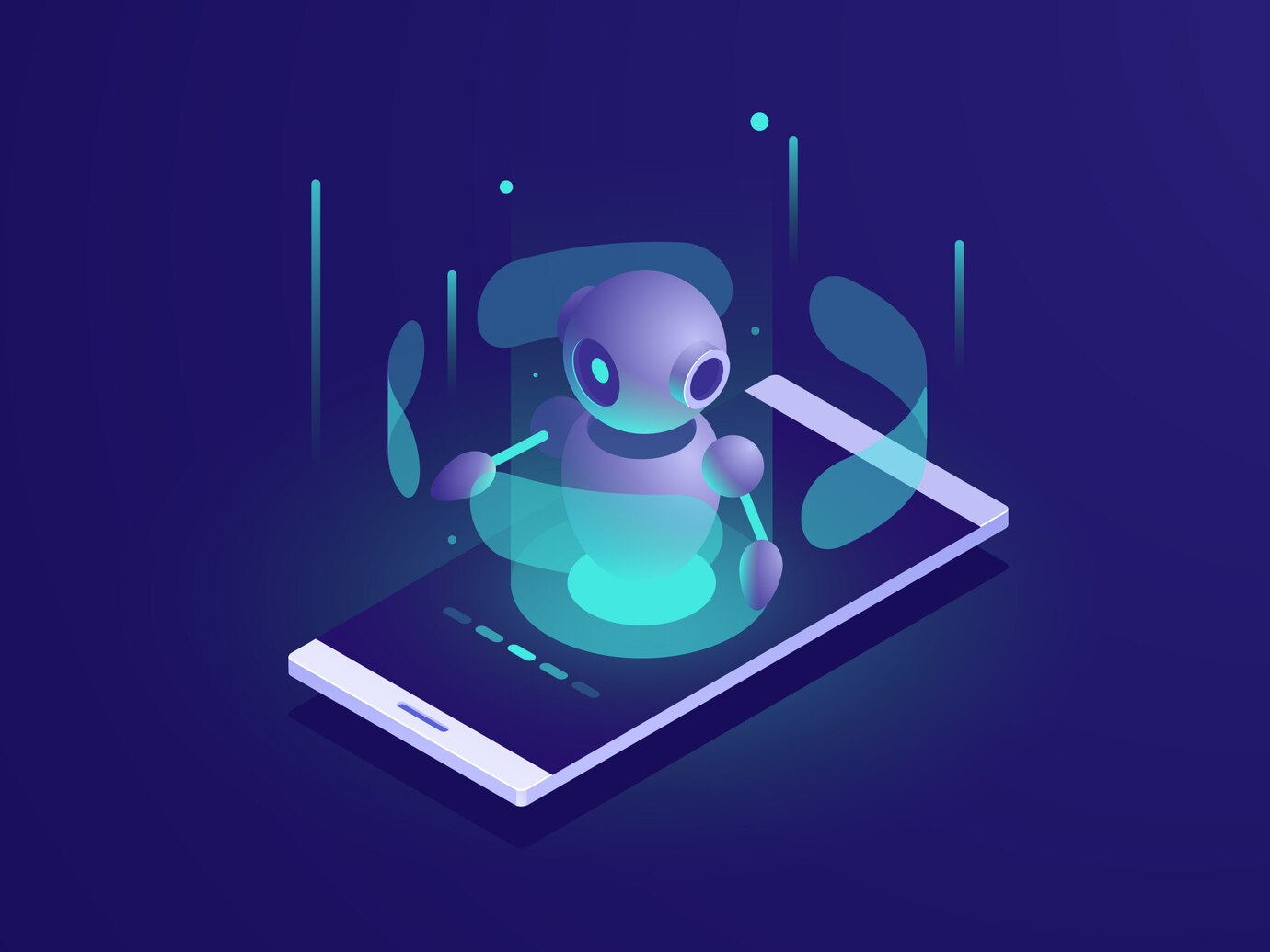
AI Applications in Banking: Real Use Cases and Industry Impact
