
How Matrix Work in Python and How to Use Them
Table of contents
Quick Access
Before getting into the topic of how to create an NXNXM Matrix in Python, we must define what a Matrix consists of within this popular programming language.
When we talk about Matrix in Python, we are referring to a specialized two-dimensional rectangular array of data, which is stored in rows and columns. Within this matrix, there can be data in the form of numbers, strings, symbols, expressions, etc. The matrix is one of the important data structures that can be used in mathematical and scientific calculations.

How does Matrix work in Python?
The data inside the matrix looks like the one shown in this graph:
First step:
Here a 2x2 matrix is shown, where two rows and two columns are observed. The data within this matrix are presented in the form of numbers, where the values 2, 3 are observed in row one and the values 4, 5 in row two. In the columns, there is column one with the values 3 and 4 and column two with the values 3 and 5.
Second step:
A slightly different 2 x 3 matrix is shown with two rows and three columns. The data within the first row has values 2, 3, 4 and in the second row, it has values 5, 6, 7. The first column has values 2.5, the second column has values 3.6 and the third column has values 4.7.
Similarly, you can have your data stored inside the nxn Matrix in Python. Many operations can be performed in addition, subtraction, multiplication, etc.
Create a Python Matrix using a nested list data type
In Python, Matrix is represented by the list data type. We are going to teach you how to create a 3x3 matrix using the list.
The matrix is made up of three rows and three columns.
- Row number one within the list format will have the following data: [8,14, -6]
- Row number two will be: [12,7,4]
- Row number three will be: [-11,3,21]
The matrix within a list with all rows and columns can look like this:
List = [[Row1],
[Row2],
[Row3]
...
[RowN]]
So, according to the above array, the Matrix's direct data list type is as follows:
M1 = [[8, 14, -6], [12,7,4], [-11,3,21]]
How we can read data into a Python Matrix when using a list
We will use the previous matrix. The example will read the data, print the Matrix, display the last element in each row.
Example: to print the matrix
M1 = [[8, 14, -6],
[12,7,4],
[-11.3.21]]
#To print the matrix
print (M1)
Production:
The Matrix M1 = [[8, 14, -6], [12, 7, 4], [-11, 3, 21]]
Example 2: Read the last element of each row.
M1 = [[8, 14, -6],
[12,7,4],
[-11.3.21]]
matrix_length = len (M1)
#To read the last element from each row.
for i in range (matrix_length):
print (M1 [i] [- 1])
Production:
-6
4
twenty-one
Example 3: to print the rows in the matrix
M1 = [[8, 14, -6],
[12,7,4],
[-11.3.21]]
matrix_length = len (M1)
#To print the rows in the Matrix
for i in range (matrix_length):
print (M1 [i])
Production:
[8, 14, -6]
[12, 7, 4]
[-11, 3, 21]
We recommend you on video
Related blogs
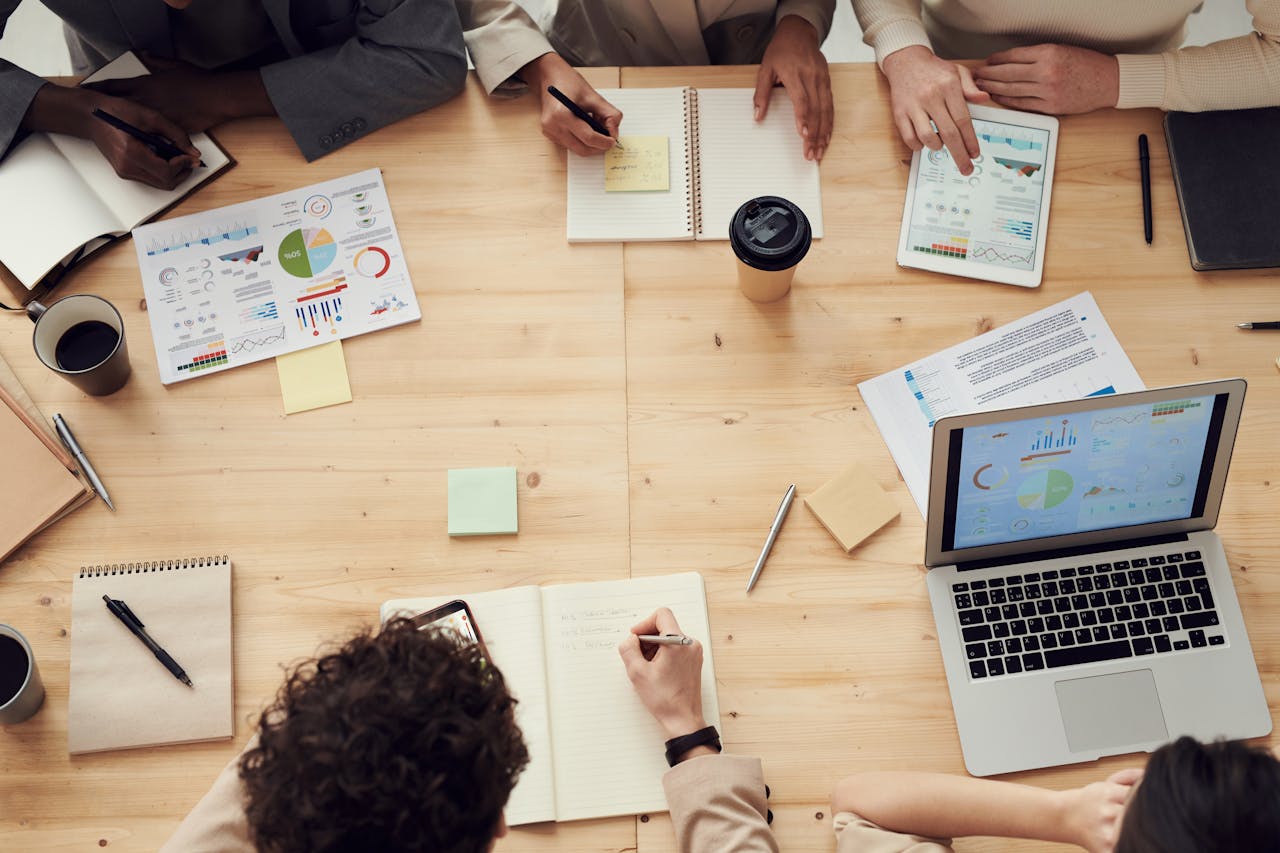
Redefining Teamwork with AI: What's New in Jira, Confluence, and Bitbucket in 2025
What is a consultation software?
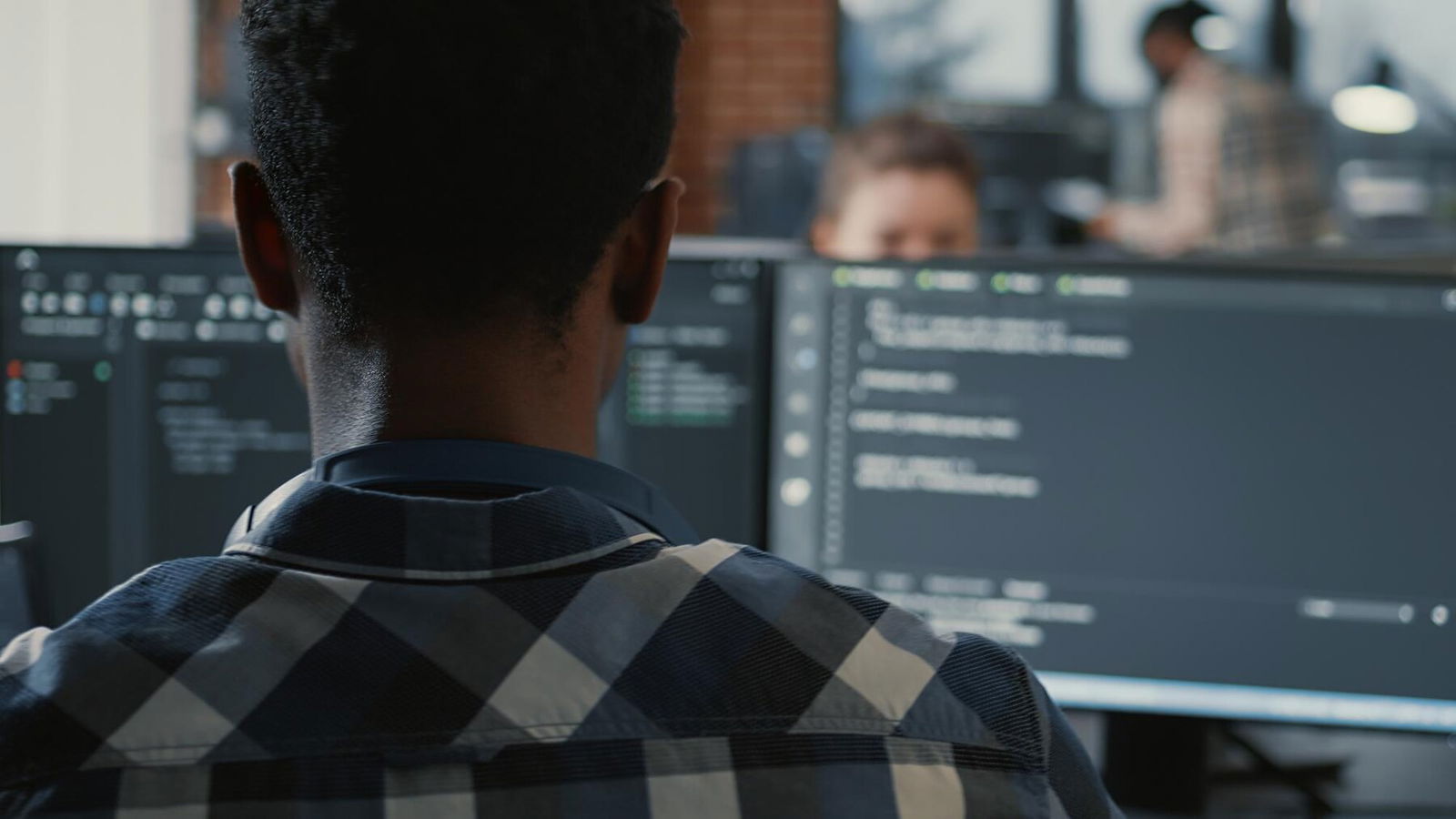
ERP Software Consultant: Rootstack is the option you need

Modern core banking: Building seamless Front-End to Back-End communication
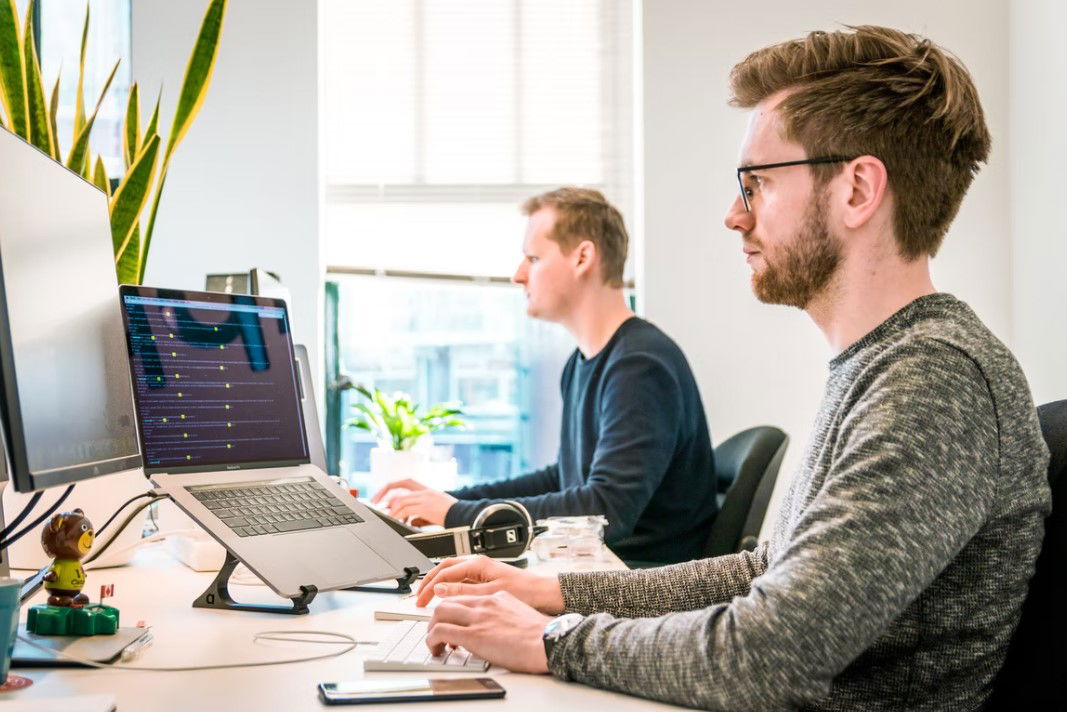
Reasons why you need a software architecture consultancy
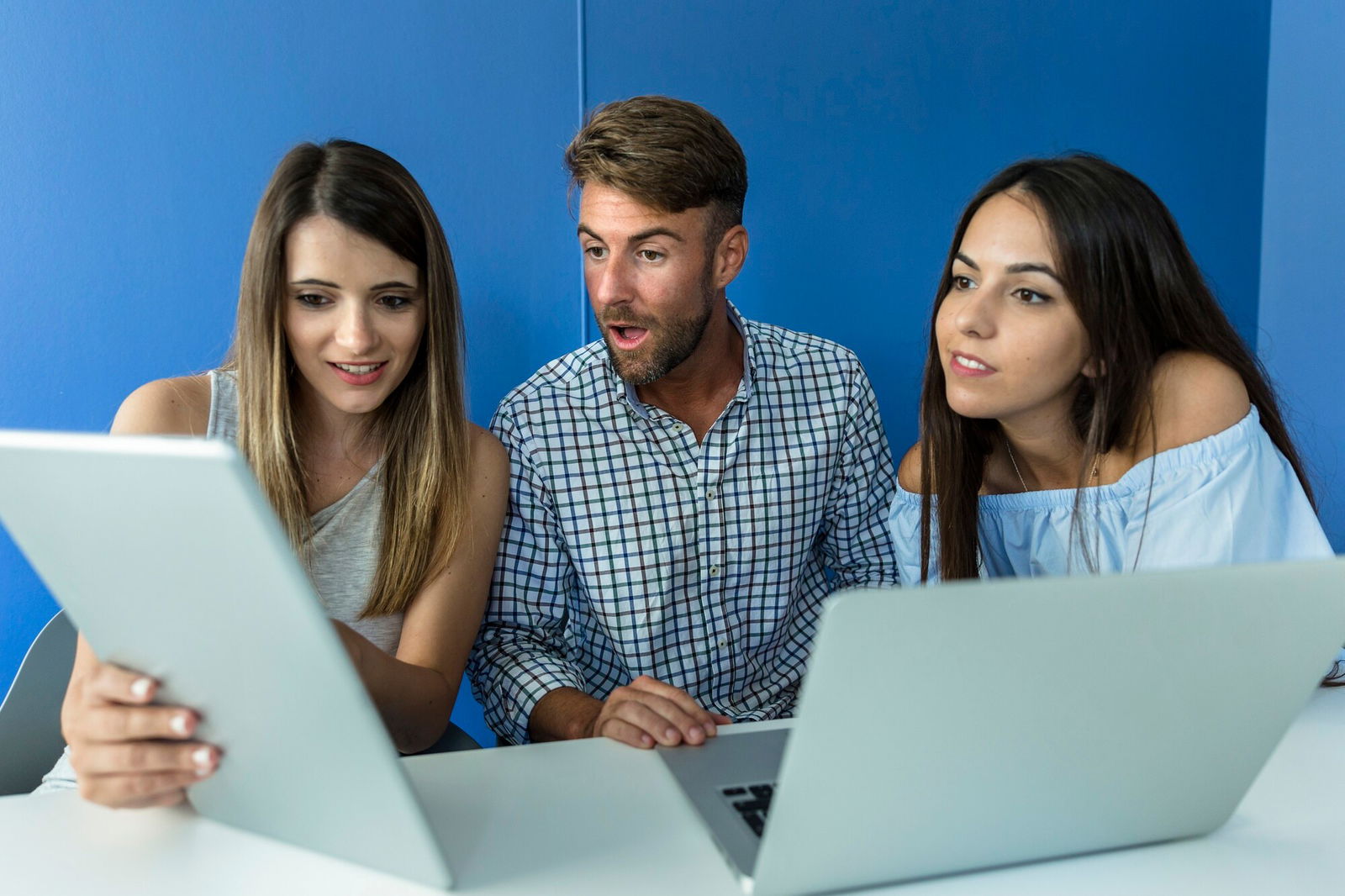