
How to Use Reactjs to Upload Files
Table of contents
Quick Access
Working with Reactjs provides various benefits to developers, including the fact that it is one of the most popular frameworks, or libraries, according to some experts, and has a large community, as well as having special functionalities that aid in the specification of any project.
ReactJS is a JavaScript open source library that is mostly used to construct user interfaces. Facebook created and maintains it, allowing developers to create fantastic online applications with dynamically updating data without having to reload the page.
ReactJS works by creating a virtual DOM (Document Object Model) that updates the real DOM only when necessary, making the process more efficient and faster. It is often used in combination with other technologies like Node.js, Redux, and webpack to build powerful and scalable applications. Read our blog about 5 tips every React developer should know.

How to upload files with Reactjs
- To begin, use the command to create a React project: create-react-app my-app npx
- In the src directory, add a new component called UploadFile.js.
- Create a file input element in the UploadFile component and add a onChange event to manage the user's file selection.
- Create a function to handle the chosen file and store it in a state variable.
- Import axios and write a function to use the axios.post() method to publish the file to the server.
- Make a formData object and add the file as a new form data field to it.
- Use the server's URL to post the formData object in the axios.post() method.
- Finally, in your main App.js file, render the UploadFile component and test the file upload functionality.
Here is an example code snippet for uploading files with Reactjs:
js import React, { useState } from 'react'; import axios from 'axios'; const UploadFile = () => { const [selectedFile, setSelectedFile] = useState(null); const handleFileUpload = (event) => { setSelectedFile(event.target.files[0]); }; const handleUpload = () => { const formData = new FormData(); formData.append('file', selectedFile); axios.post('/api/upload', formData) .then((response) => { console.log(response.data); }) .catch((error) => { console.log(error); }); }; return( <div> <h3>Upload File</h3> <input type="file" onChange={handleFileUpload} /> <button onClick={handleUpload}>Upload</button> </div> ); }; export default UploadFile;

Importance of uploading files with Reactjs
Uploading files with Reactjs can improve the user experience by making it simple and straightforward. It also connects easily with other technologies such as axios, which aids in sending the file to a server.
Developers can use Reactjs to add additional features to the file upload process, such as progress bars that display upload status in real time. It is also feasible to incorporate security measures to ensure that submitted files are safe and only accessible to authorized users.
In general, using Reactjs to upload files is vital for improving the user experience, making file uploads more efficient, and adding sophisticated functionality to the process.
Learn more about Building a Social Media App with React: A Complete Tutorial. Let's work together!
Other uses of Reactjs apart from UI development
Reactjs is an open source JavaScript library used to create user interfaces. It is widely adopted by developers due to its ability to render dynamic and interactive user interfaces. Besides building user interfaces, here are some other uses for Reactjs:
- Single Page Apps: Reactjs may be used to create quick and dynamic single page apps that do not necessitate a page reload with each user activity.
- Mobile Apps: React Native is a Reactjs-based framework used to create mobile apps that are indistinguishable from traditional native apps.
- Static Websites: Gatsby is a static site builder built using Reactjs. It allows you to build static webpages with the help of Reactjs and GraphQL.
- Server Side Rendering: Reactjs can be used for server side rendering to improve search engine optimization, boost performance, and allow search engines to scan dynamically rendered client-side content.
- Desktop Applications: Using frameworks such as Electron, Reactjs may be used to create cross-platform desktop applications.
- Component Libraries: Reactjs allows developers to create reusable UI components that can be shared and used across many projects.
- Game Development: Several game engines, such as React Game Kit, are developed on top of Reactjs and allow developers to easily create games using Reactjs components.
Reactjs is a versatile and powerful library that can be used to build various types of applications and interfaces.
We recommend you on video
Related blogs
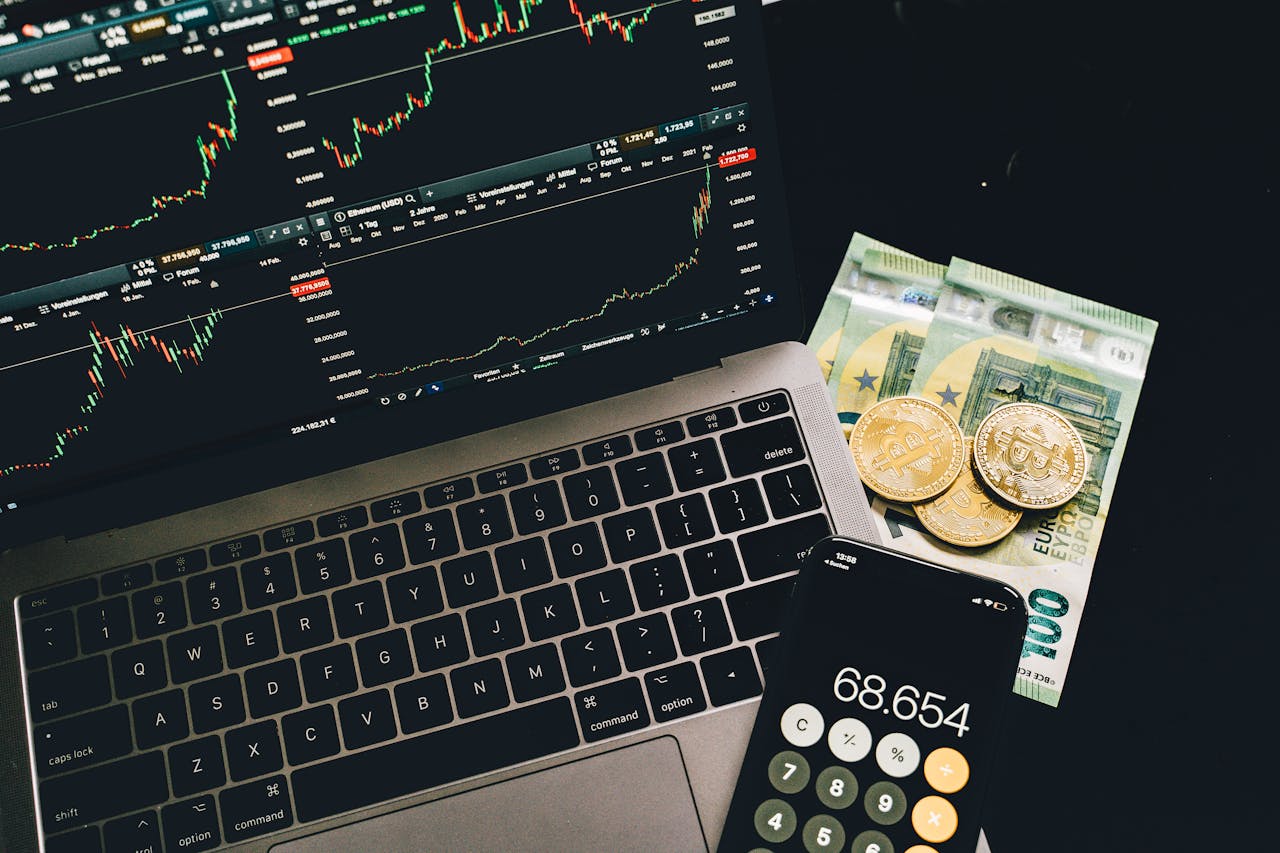
Portfolio management and collection software for rural areas of Panama
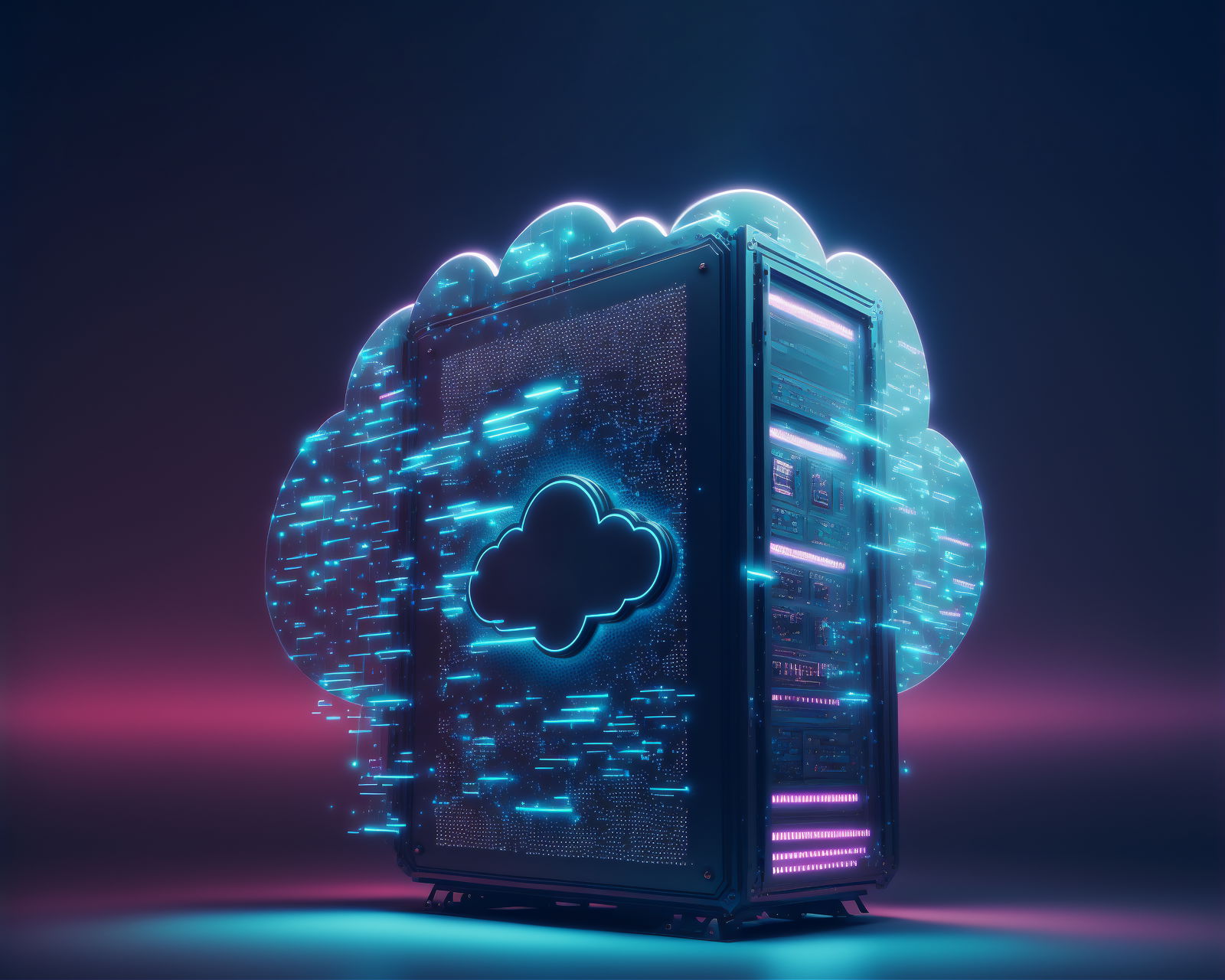
Q Business Suite: The future of the workplace AI in the cloud, combined with QuickSight and Q Apps
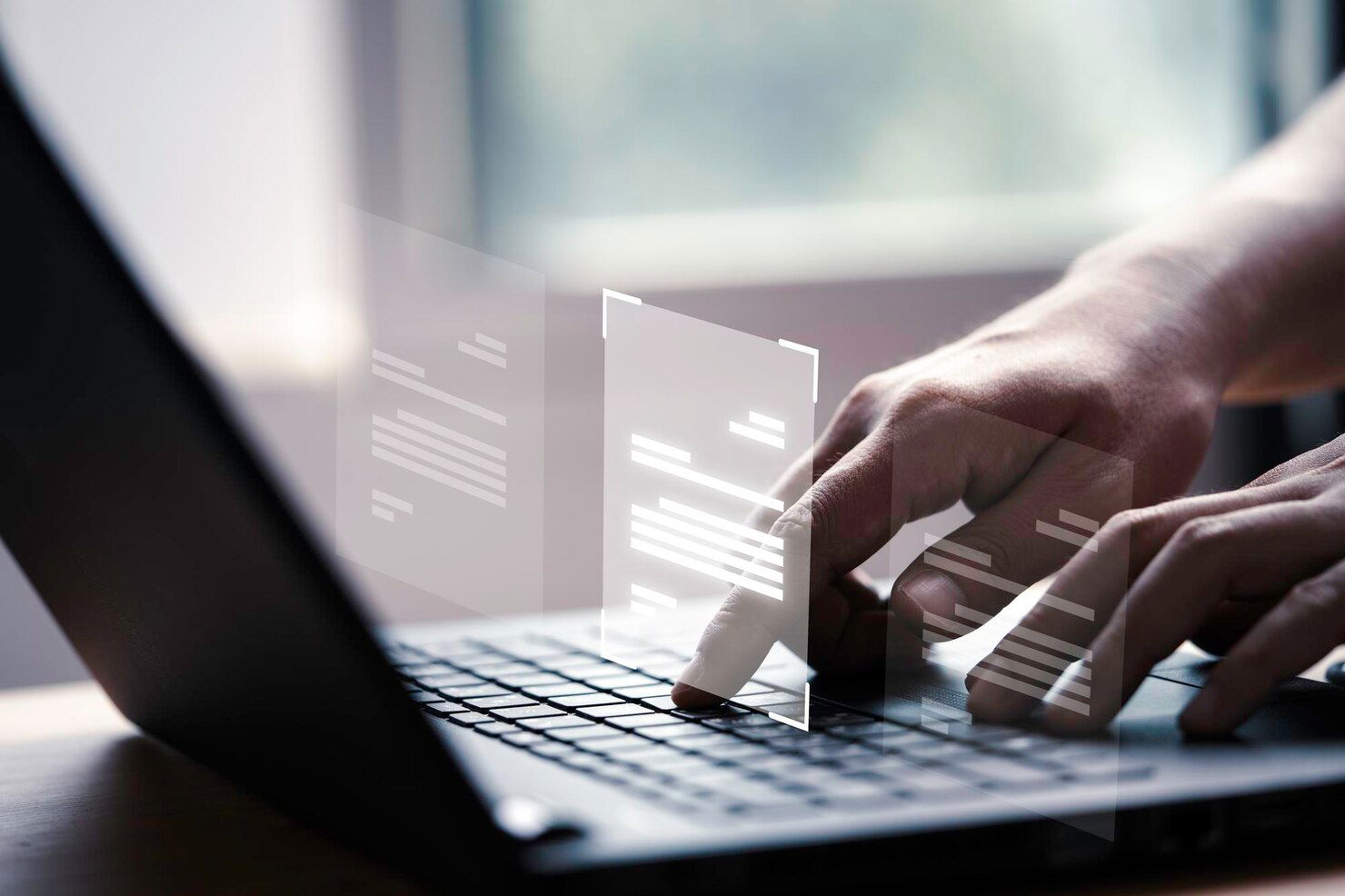
What's new in Odoo 18: from roadmap to action
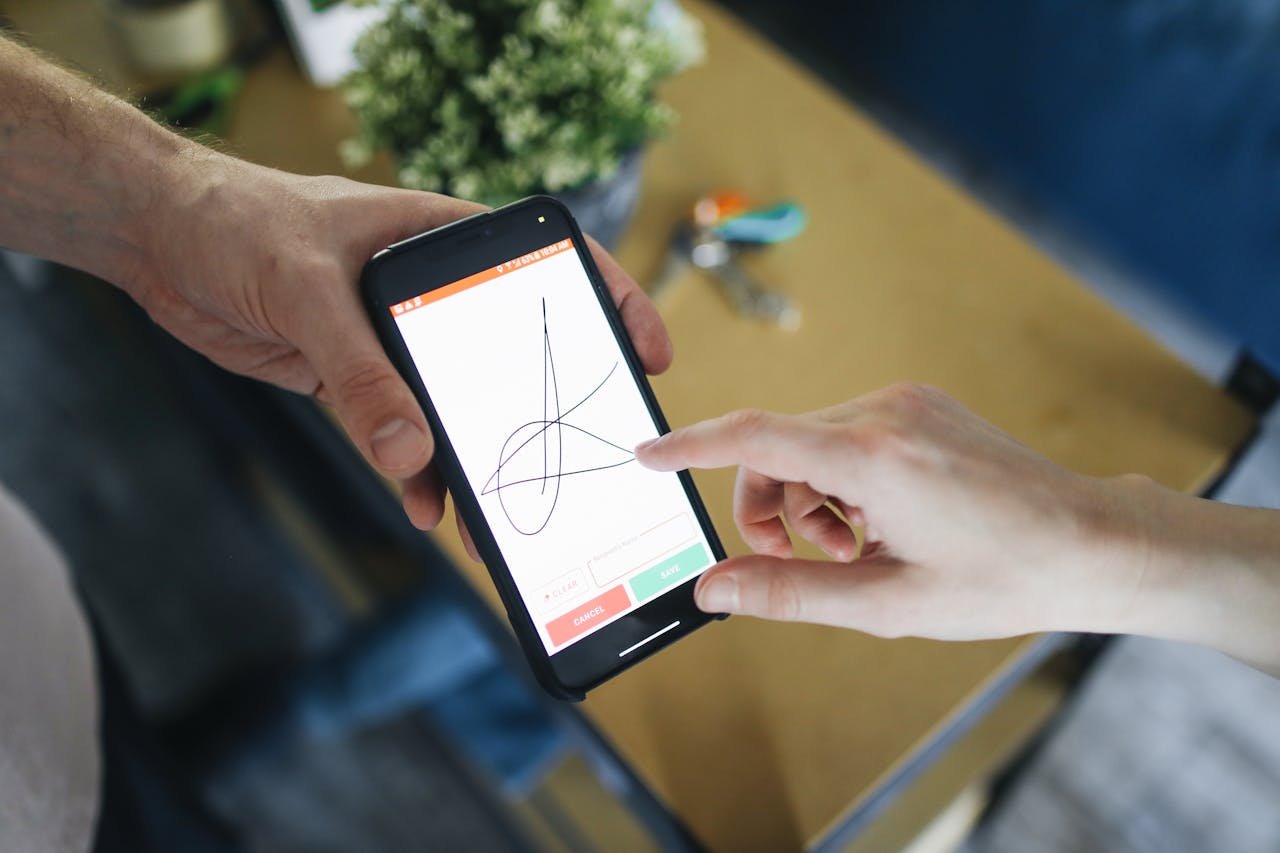
Key developments in Validated ID in 2025
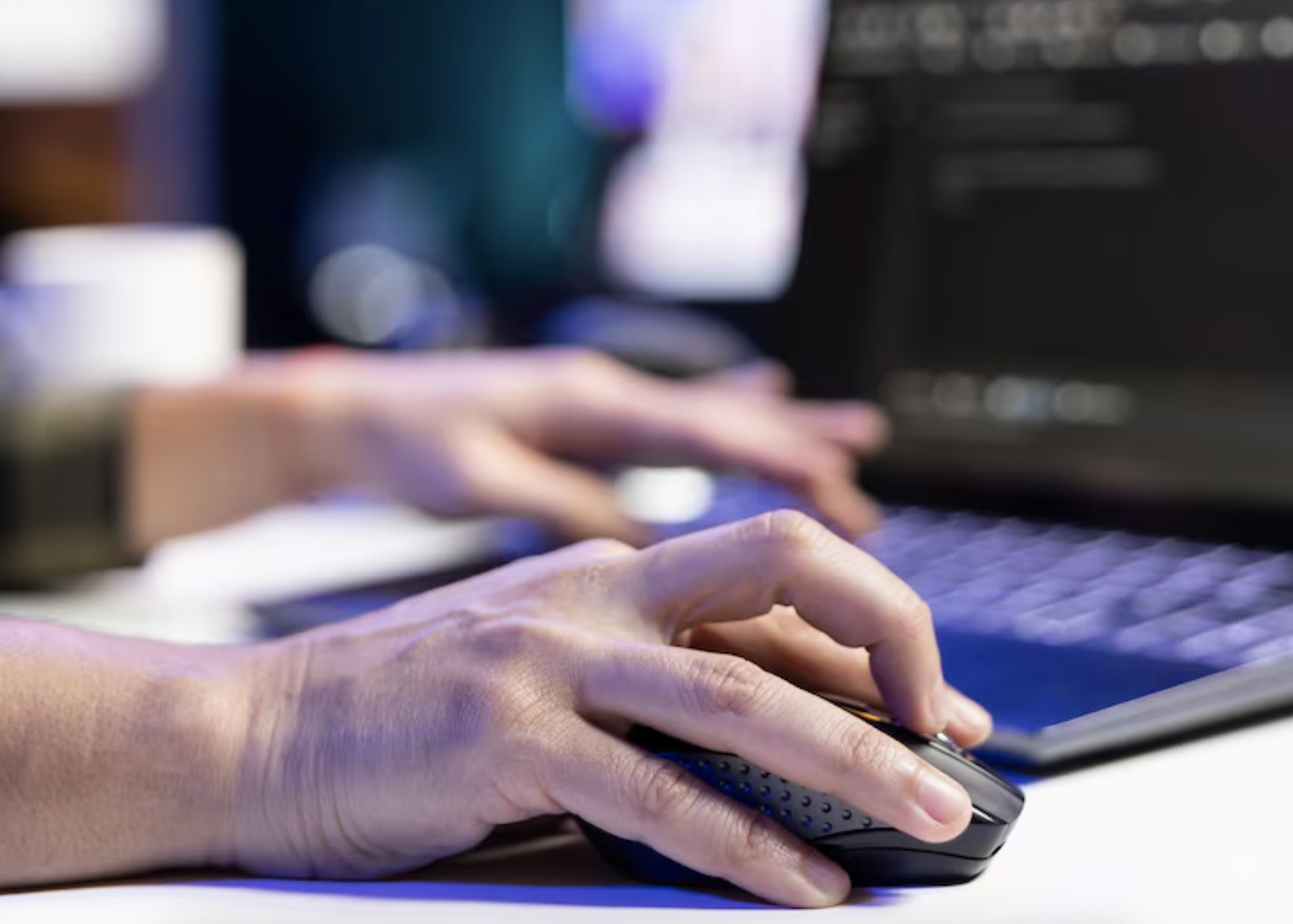
Software unit testing companies in Colombia: Rootstack your best option
