
Paradigms supported in Python
Table of contents
Quick Access
There are 4 programming paradigms used in Python, each has its benefits and uses depending on the situation. Python web frameworks, for example, typically use a mix of procedural and object-oriented paradigms.
Object-oriented paradigm
It is one of the most common paradigms, it uses the concepts of classes, objects and inheritance, among others, to structure the code. Python allows inheritance, which means that one class can inherit attributes and methods from another.
# Creación de clase class Persona: # Método de inicialización def __init__(self, nombre, edad): self.nombre = nombre self.edad = edad # Método def mostrar_informacion(self): print(f"Nombre: {self.nombre}, Edad: {self.edad}") # Creación de objetos de clase personas con valores iniciales persona1 = Persona("Juan", 30) persona2 = Persona("María", 25) # Ejecución de métodos de cada objeto persona1.mostrar_informacion() persona2.mostrar_informacion()
Procedural paradigm
The procedural paradigm in Python performs a sequence of execution of different functions that encapsulate blocks of code that perform specific tasks, organizing them through loops and other control structures such as conditionals.
# Creation of function (it does not belong to any class) def calculate_average(numbers): sum = 0 for number in numbers: sum += number average = sum / len(numbers) average return numbers = [5, 10, 15, 20, 25] # Function call (note that it does not belong to any object) average = calculate_average(numbers) print(f"The average of the list is: {average}")
Functional paradigm
In the functional paradigm, as the name says, the main unit is the functions; making great use of lambdas, `map()`, `filter()` and `reduce()`. This approach promotes the use of simple functions, without side effects; avoiding the use of conditionals or complex loops that modify the data and replacing them with multiple simpler functions that connect together.
from functools import reduce numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] def square(number): return number ** 2 def is_even(number): return number % 2 == 0 def sum(a, b): return a + b # Using map() to square each number squares = list(map(square, numbers)) # Using filter() to get even numbers even_numbers = list(filter(even_numbers, numbers)) # Using reduce() to add all the numbers sum_total = reduce(sum, numbers) print("Square:", square) print("Even numbers:", even_numbers) print("Total sum:", total_sum)
Imperative paradigm
Technically procedural programming is also imperative, however, in this case we are referring to the explicit calling of instructions that can be given on the data. A common use is to analyze data such as in a Jupyter Notebook or directly execute commands in the Python console.
Language Concepts
Dataclasses
They are classes that are created for convenience with the primary purpose of storing data. These include by default special methods for comparing values and representing them as strings.
from dataclasses import dataclass # Creation of a dataclass. No need for a __init__ @dataclass class Person: name: str age: int # Object creation person1 = Person("John", 30) person2 = Person("Mary", 25) # Direct access to attributes print(person1.name) print(person2.age) # Display the object as __repr__ print(person1) # Object comparison via __eq__ print(person1 == person2)
Lambdas
Lambda functions are widely used in functional programming; this functionality allows creating anonymous functions, commonly hosting simple and short operations.
# Definition of a lambda function sum = lambda x, y: x + y result = sum(3, 5) print(result) # It is also possible to use them directly numbers = [1, 2, 3, 4, 5] squares = list(map(lambda x: x ** 2, numbers)) print(squares)
Decorators
Decorators are functions that modify or wrap other functions or methods (such as the dataclass that creates special methods on the class). They are used to extend functionality of functions or alter their behavior.
# Definition of a decorator def wrapper_decorator(func): def message(): print("Before calling the function.") func() print("After calling the function.") return message @wrapper_decorator def greeting(): print("Hello, world!") # Decorated function call greeting()
Tips: How to get familiar with the code easier
- General view of the project structure, either from the IDE or using the `tree` command to identify if there are directories such as `models`, `routes` (focused mostly on the web) or `services`.
- Review at least one of the files in each of these directories to, apart from knowing their location, the role they play within the project. Example, how much logic is being abstracted into services.
- Once familiar with these files, it is easier to review the source code of the "main" file (it may vary if it is CLI, a lambda, a framework) since without needing to know the internal code of each file or method, we can follow the logic knowing the role of each of the classes.
We recommend you on video
Related blogs
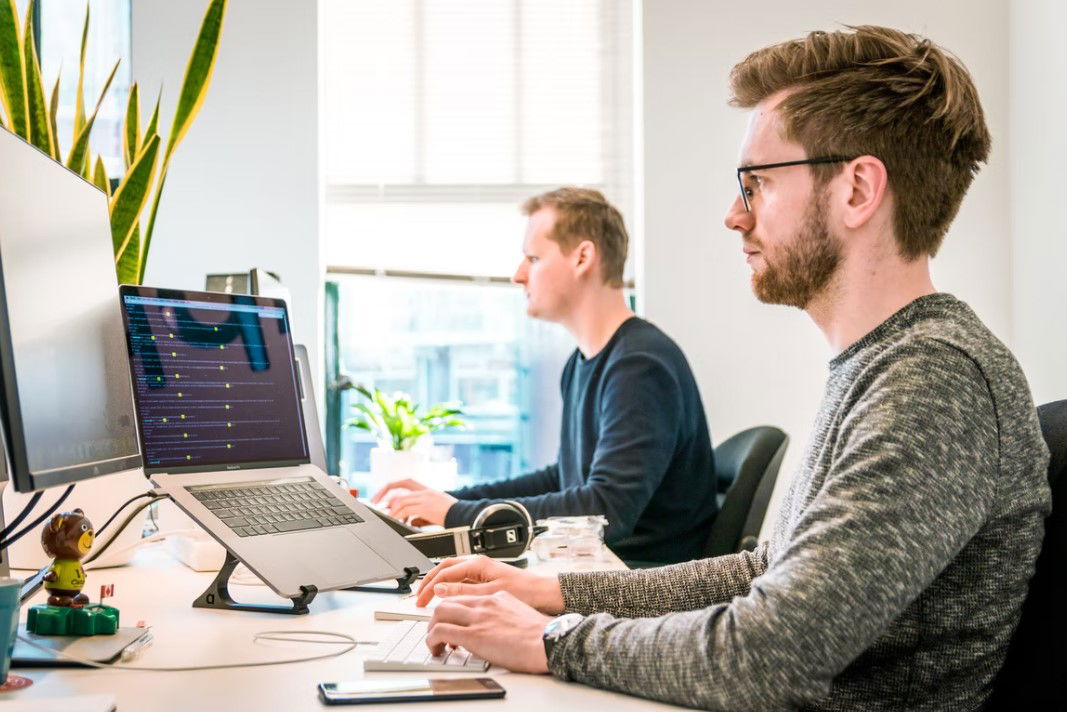
Reasons why you need a software architecture consultancy
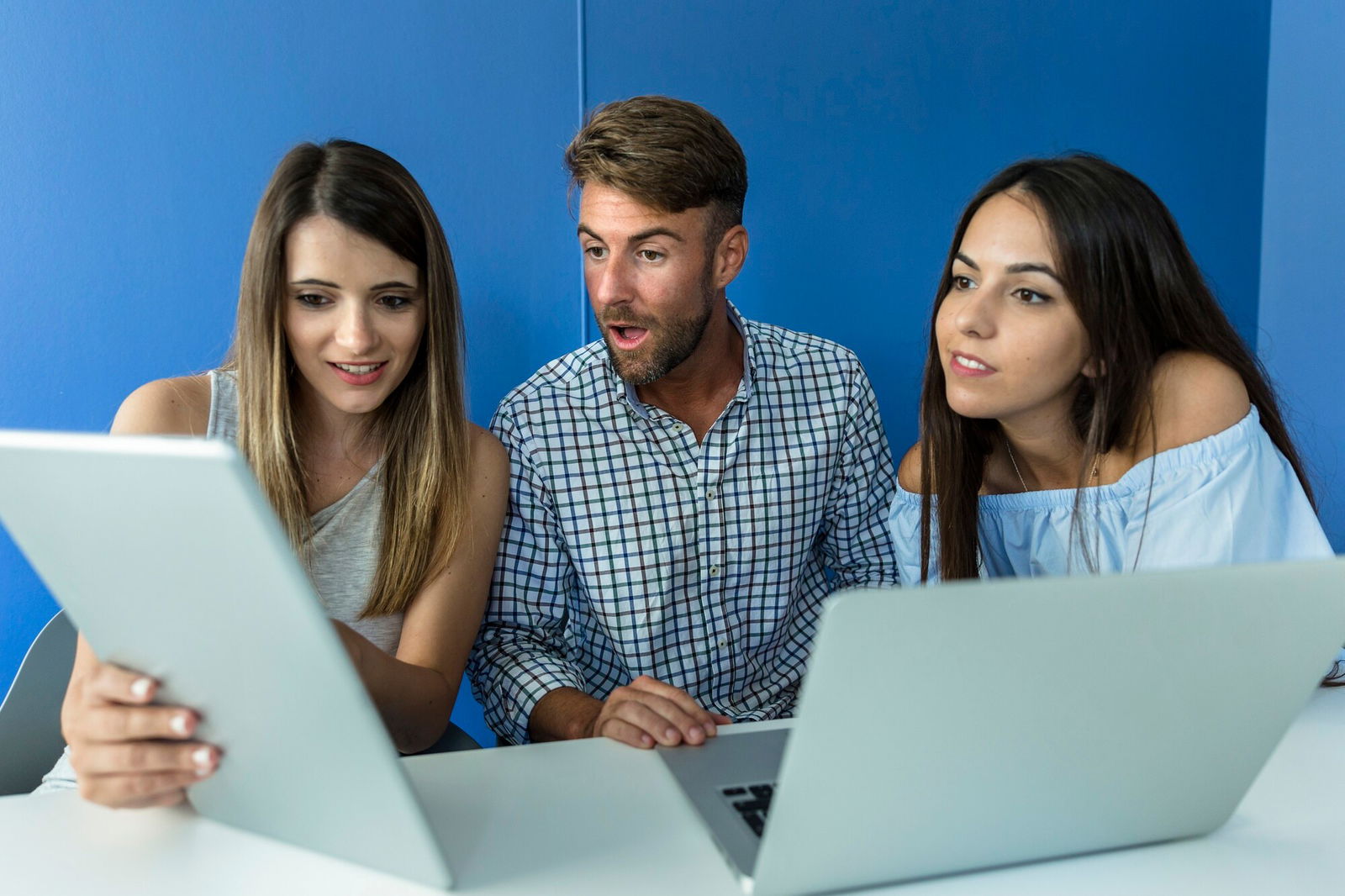
Top-tier software consulting services: Rootstack is your best choice

Blacklists, biometrics, and banking: Automating risk verification in the age of AI
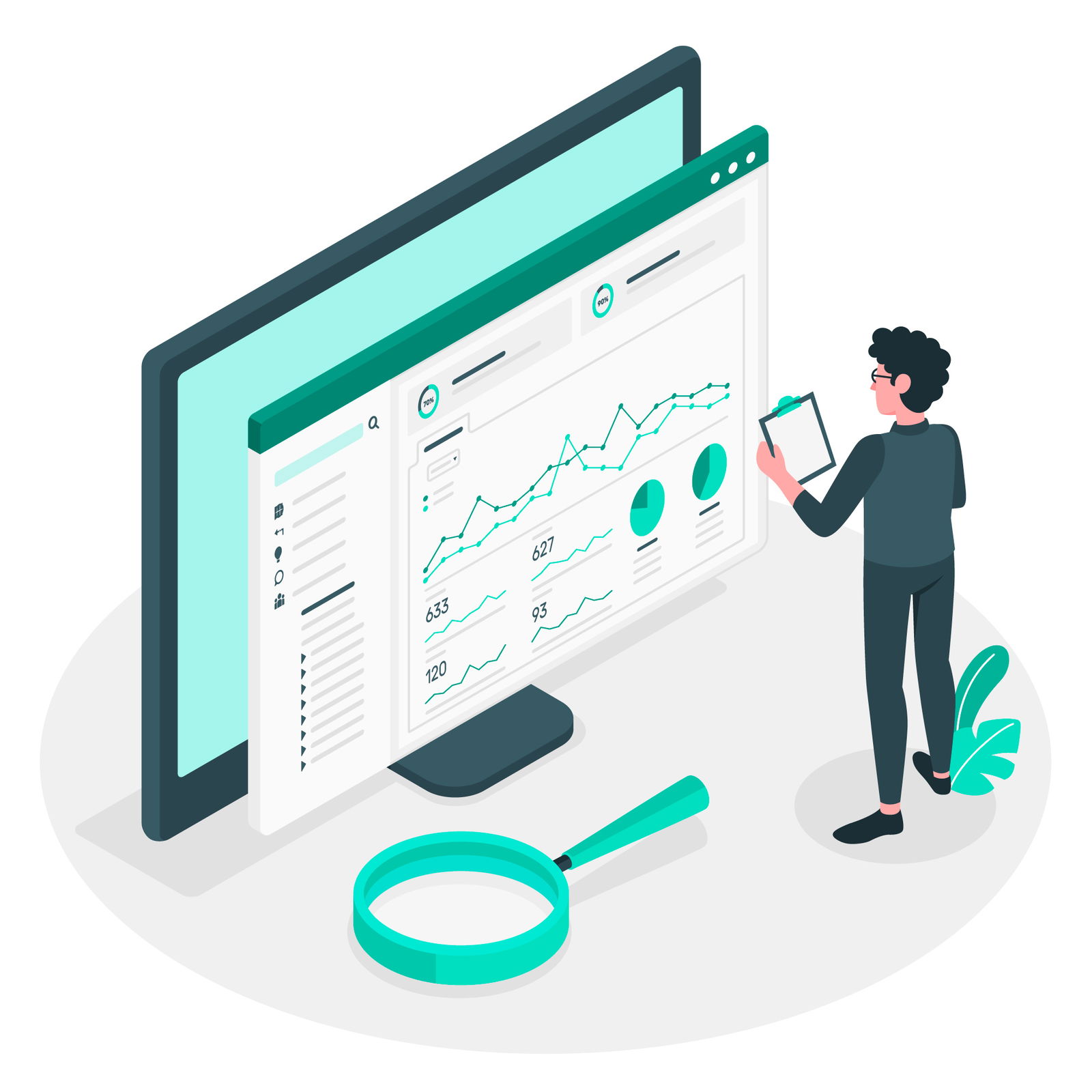
From Excel to Intelligence: Why banks must centralize data to compete
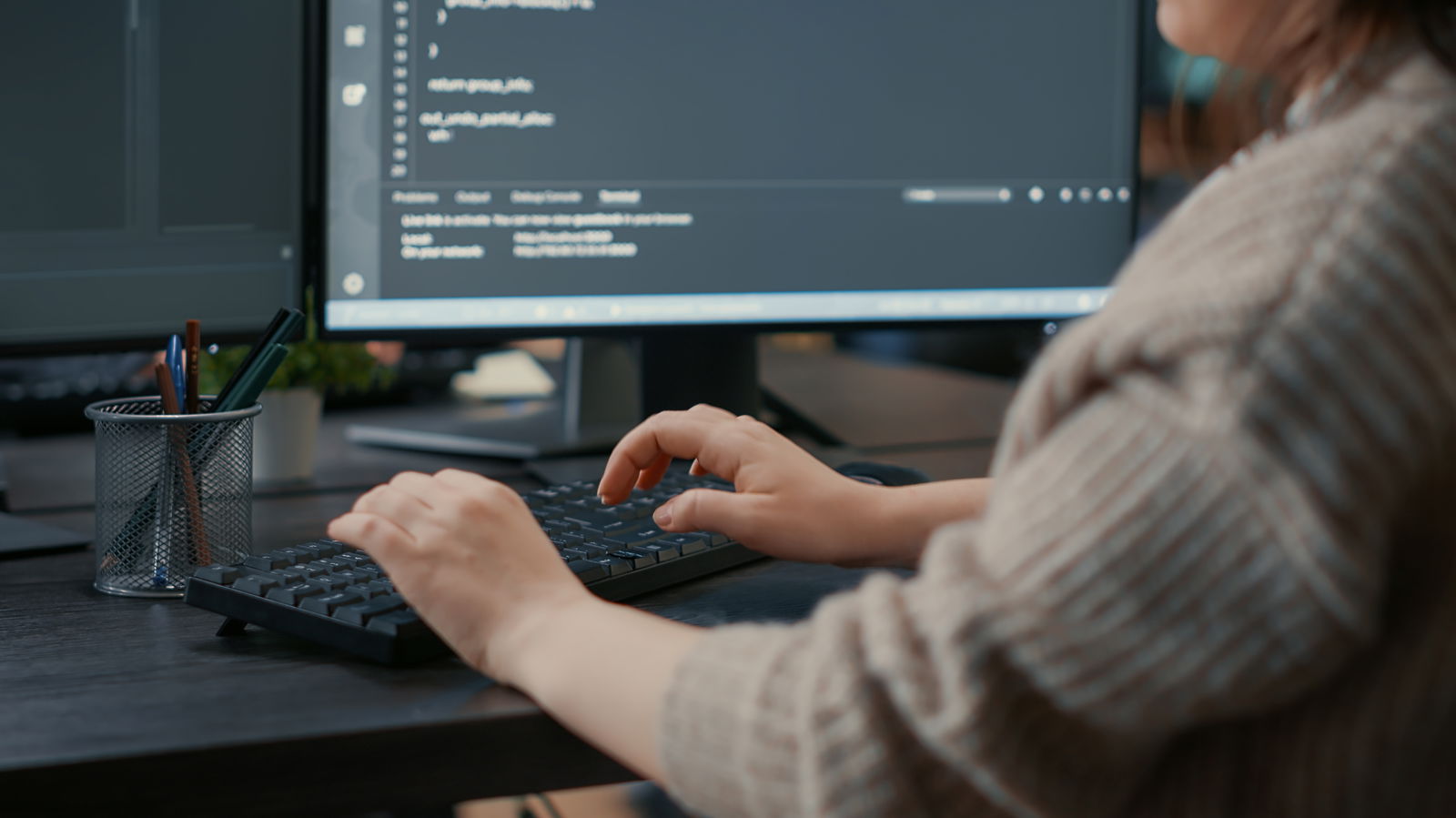
How to Choose the Best Software for Risk Modeling in 2025
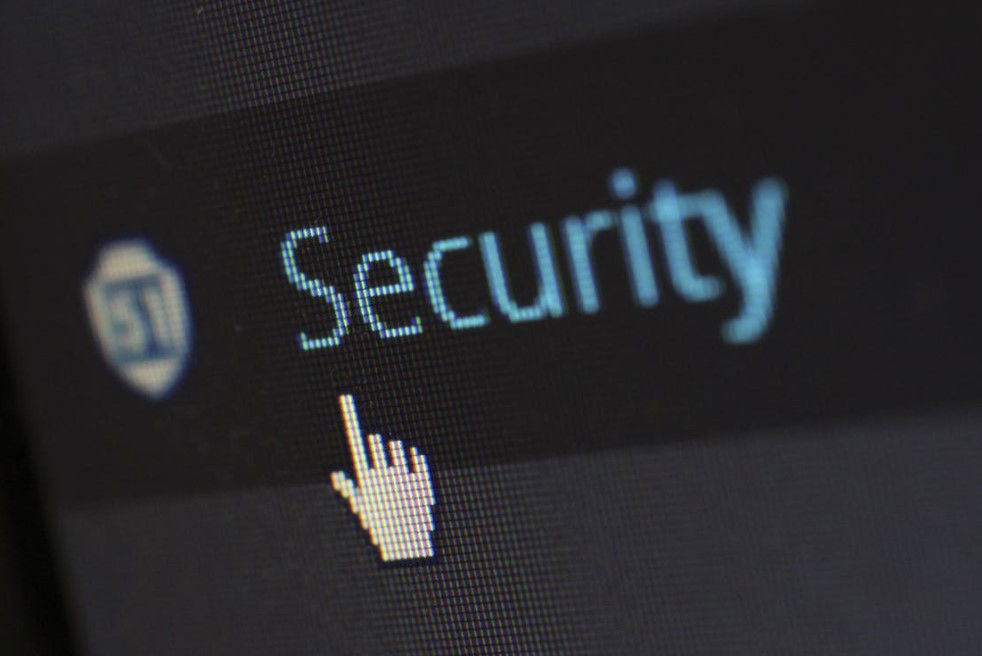